Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial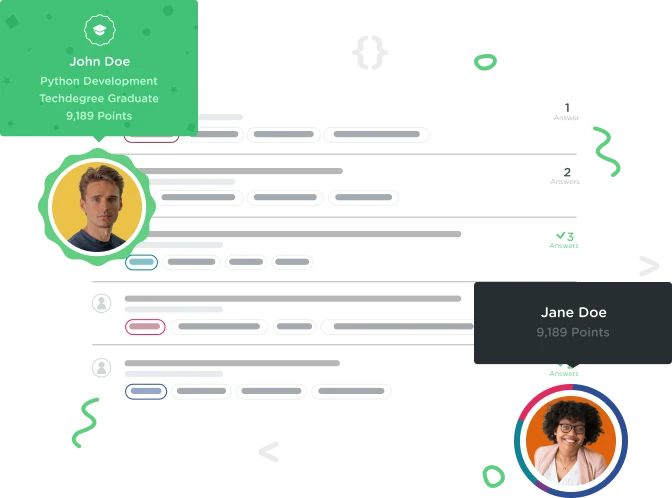

Pratham Patel
4,976 Pointscan I have the answer to this problem
I don't see it
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
lapsDriven += laps;
if(laps > MAX_BARS) {
throw new IllegalArgumentException();
}
barCount -= laps;
}
}
2 Answers

andren
28,558 PointsThere are two issues with your code:
The if statement should be placed at the top of the function, you should not increase
lapsDriven
before you potentially throw the exception.You compare the requested laps to
MAX_BARS
. TheMAX_BARS
variable holds the maximum charge the GoKart can store, not the current charge it actually has. The current charge is stored inbarCount
.
If you fix those two issues like this:
public void drive(int laps) {
if(laps > barCount) {
throw new IllegalArgumentException();
}
lapsDriven += laps;
barCount -= laps;
}
Then you'll be able to pass the challenge.
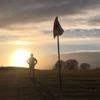
Stuart Wright
41,120 PointsIn your if statement, you need to compare laps to barCount rather than MAX_BARS. Because the kart might not be at max battery when it receives the drive request. With that one change, your code should pass.
Edit: I see that Andren beat me to it. Although I agree with point 1 above, it seems that's not actually necessary to pass the challenge.