Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial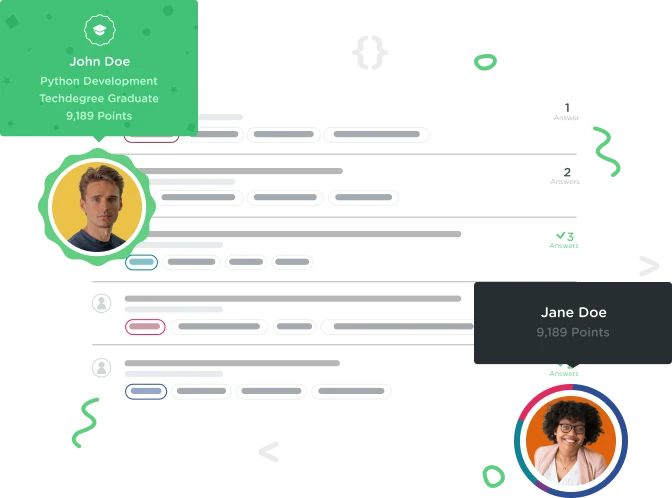
1 Answer

martinjones1
Front End Web Development Techdegree Graduate 44,824 PointsGood stuff, it looks really good what you are looking to achieve.
Code can always be improved and the improvements can be quite subjective to each individual and team.
I have included some changes below that you may be of interest, there are still improvements that can be made, but hopefully this will help and give some ideas
To summarise, I approached it with the ideas below in mind, not all of them related to the function exactly
- created a new validateUserInput function to help keep the code DRY
- removed the else clause after the if statement since you would always return and break out of the if statement anyway
- changed the spelling of "lenhgt" to "length" (this could make it easier to do a CTRL + f find for this string)
- formatted the code indentation
- moved the code below each comment to make it easier for me to see how the two related (save scrolling up and down)
// 1. Attach this file geometry.js to the index.html file
function validateUserInput(userInput) {
if (isNaN(userInput)) {
alert("You put a letter or a world in width or height");
}
}
// 2. Create a function that calculates the area of a rectangle.
// The function should accept the width and height as arguments
// and return the area of that rectangle.
// The area of a rectangle is the width * height
// this will give the user the area of a rectangle
function rectangleArea(width, height) {
var width = parseFloat(prompt("What is the width?"));
var height = parseFloat(prompt("What is the height?"));
if (validateUserInput(width) || validateUserInput(height)) {
return rectangleArea();
}
return Math.round(width * height * 100) / 100;
}
//console.log( rectangleArea() );
// 3. Create a function that calculates the volume of a rectangular prism.
// The function should accept the width, height and length as arguments
// and return the volume of that rectangular prism.
// The volume of a rectangular prism is the width * height * length
// this will give the user the prism of a rectangle
function rectangularPrism(length) {
var length = parseFloat(prompt("What is the length?"));
if (validateUserInput(length)) {
return rectangularPrism();
}
return Math.round(rectangleArea() * length * 100) / 100;
}
//console.log( rectangularPrism() );
// 4. Create a function that calculates the area of a circle.
// The function should accept the radius of the circle as an argument
// and return the area of that circle.
// The area of a circle is the value of π * radius^2
// this will give the user the circle area
function circleArea(radius) {
var radius = parseFloat(prompt("What is the radius?"));
if (validateUserInput(radius)) {
return circleArea();
}
return Math.round(Math.PI * Math.pow(radius, 2) * 100) / 100;
}
//console.log( circleArea() );
// 5. Create a function that calculates the volume of a sphere.
// The function should accept the radius of the sphere as an argument
// and return the volume.
// The volume of a circle is: 4/3 * π * radius^3
// this will give the user the volume of a sphere
function circleVolume(radius) {
var radius = parseFloat(prompt("What is the radius?"));
if (validateUserInput(radius)) {
return circleVolume();
}
return Math.round((4 / 3) * Math.PI * Math.pow(radius, 3) * 100) / 100;
}
//console.log( circleVolume() );
// 6. Use console.log to test each function and output to the JavaScript console
// Here are the values to test and the expected results
// -- Area of rectangle that is 5 wide and 22 tall: 110
// -- Volume of a rectangular prism that is 4.5 x 12.5 x 17.4: 978.7499999999999
// -- Area of a circle that with a radius of 7.2: 162.8601631620949
// -- Volume of a spehere with a radius of 7.2: 1563.4575663561109
jay aljoe
4,113 Pointsjay aljoe
4,113 Pointswhat function? could you post the function and explain what it does?