Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial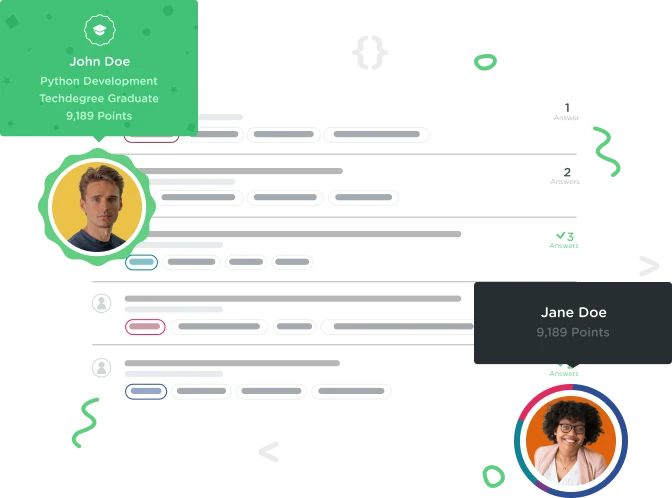
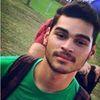
Gustavo Winter
Courses Plus Student 27,382 PointsCan i override a method in JavaScript as like in Java?
I would like to know, if it's possible to override a function in JavaScript.
Example:
function example(job){
console.log("My job is ${job}");
}
hypothetically, in the future if this person above, get another job could i override the function example, to add the new job and keeping the previous.
I guess, what i try to saying is just to add this line.
console.log("Now i have two jobs, and my new job is ${newJob}");
More and less like the override in Java Language.
2 Answers
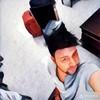
Ari Misha
19,323 PointsHiya there! Arent ya over-riding everything everytime? As soon as you type the keyword function you're overriding whether its Ruby or Python, or Java or JavaScript. It mostly happens in Object-oriented programming languages. And Java is the best example of it. So is Ruby , C# and Python. Everything we type already defined for us in higher hierachy in the Language itself.
JavaScript is weird when coming from other languages like it lets add and multiply int with strings. I know thats crazy right? Also JS has something prototypal inheritance right? Which looks even more weird confusing. But thats the power of JavaScript which is eating the IT right now.
~ Ari

Alexander La Bianca
15,959 PointsYes you can. However, you are not overriding it in the sense like you are in Java. That is because javascript uses prototypal inheritance. Not an expert in Java, but overall with object oriented programming you'd like to override a method in a subclass of some sort. So that the overridden method performs some sort of specific action. In Java I believe you use the @Override annotation?
In javascript this is much simpler using prototypal inheritance
//The Super 'Class' Person
function Person(name,age) {
this.name = name;
this.age = age;
}
//has a method -- introduce
Person.prototype.introduce = function() {
console.log("Hello I am " + this.name + " and I am " + this.age + " years old");
}
//The subclass SoftwareTester - inherits the prototype from Person
function SoftwareTester(name,age) {
Person.call(this,name,age);
this.profession = "Software Tester";
}
SoftwareTester.prototype = Object.create(Person.prototype);
//Now here is where i 'override' the introduce method from my Person class. I simply add an introduce method to the SoftwareTester class prototype
SoftwareTester.prototype.introduce = function() {
console.log("Hello I am " + this.name + " and I am a " + this.profession);
}
const person = new Person("Ashley", 25);
const tester = new SoftwareTester("Alex", 24);
person.introduce();
tester.introduce();
It may be important to explain why this works. If you console log, both the person and the tester, then you will see their prototype properties. The tester has two prototype properties since it inherited one from Person. When I do tester.introduce() javascript goes along the prototype chain. If it can find introduce() in the prototype of tester, then it will use that one (which is the case here). If it does not find introduce() in the tester prototype, it goes up one level. Which would be the prototype for Person. There introduce() exists as well. Which we would have used if introduce() did not exist on a tester level.
Hope that makes sense.