Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial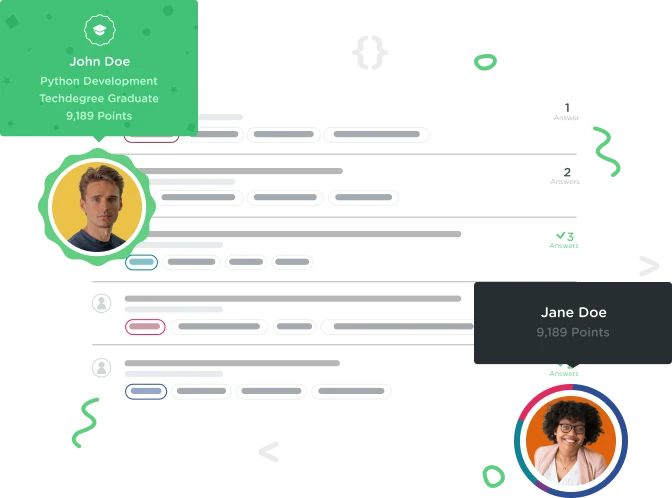

Pilar Fernandez
3,085 PointsCan I select child elements of a tag name in JS? i.e selecting the li elements of a ul that is under a nav
I'm learning how to select elements and I was wondering if it's possible to select child elements of one or more tag names. For example: document.getElementByTagName('nav > ul > li') - I know this isn't the right code, but it "explains" what I'm trying to do.
let navigationLinks = document.getElementByClassName('nav');
let galleryLinks;
let footerImages;
let navigationLinks
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Nick Pettit | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Nick Pettit</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="index.html" class="selected nav">Portfolio</a></li>
<li><a href="about.html" class="nav">About</a></li>
<li><a href="contact.html" class="nav">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="img/numbers-01.jpg">
<img src="img/numbers-01.jpg" alt="">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="img/numbers-02.jpg">
<img src="img/numbers-02.jpg" alt="">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="http://twitter.com/nickrp"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="http://facebook.com/nickpettit"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© 2016 Nick Pettit.</p>
</footer>
</div>
<script src="js/app.js"></script>
</body>
</html>
2 Answers

andren
28,558 PointsBy using the querySelector
and querySelectorAll
methods you can select elements using any CSS selector. I don't know if you have any CSS experience but CSS has a myriad of selectors and they are quite flexible, enabling you to select elements in pretty much any way you can imagine.
The type of selector you are looking for is the descendant selector which selects elements only if they are a descendant of the other elements you specified.
The descendant selector is pretty simple, in fact it's pretty similar to the example selector you wrote. You just have to type the element names with just a space between them. Like this:
let navigationLinks = document.querySelectorAll('nav ul li');
That will select li
elements that are under a ul
element which itself is under a nav
element. Descendant selectors don't have to be that specific though, you don't have to specify every step of the chain. You can for example just shorten the selector to this:
let navigationLinks = document.querySelectorAll('nav li');
Though one thing I would point out is that the task you are currently working on asks you to select links that are found under a navigation element. li
elements are not links, they are list-elements. The tag that is used to create links is a
.
So the selector that will find the link elements this task asks for is this:
let navigationLinks = document.querySelectorAll('nav a');
If you are curious about learning more about CSS selectors then I would recommend this article as it lists some of the most useful selectors available.

Pilar Fernandez
3,085 PointsThank you both!!
andren: Yes, I'm very familiar with CSS. I just wasn't sure how descendant selectors work with JS. As far as I remember i tried with single space, colon and dash.. and nothing worked. I probably made a typo though. Thank you so much again! This really helps :)
Amber Fuller
4,598 PointsAmber Fuller
4,598 PointsYes, it is possible. I've found a link to the MDN on it https://developer.mozilla.org/en-US/docs/Web/API/ParentNode/children. However, it's not supported by all browsers according to MDN.