Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial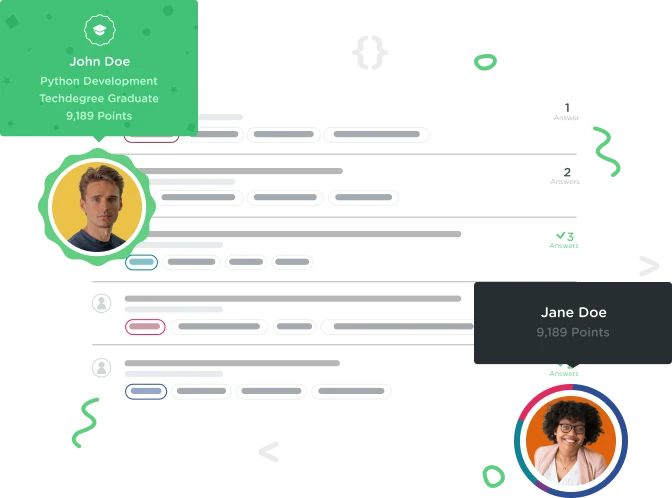

Kayc M
12,593 PointsCan I use a forEach loop instead?
I tried this but it won't work
createTokens(num) {
const tokens = new Array(num);
tokens.forEach((token) => {
new Token(this);
tokens.push(token);
});
return tokens;
}
5 Answers
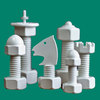
Steven Parker
230,274 PointsWithout knowing anything about what this is supposed to do, this stands out:
new Token(this); // <-- this creates an object, but doesn't save it anywhere
tokens.push(token); // <-- did you mean "tokens.push(new Token(this))" instead?
To allow a more thorough analysis and complete answer, please provide a link to the page you are working with and if possible, make a snapshot of your workspace and post the link to it here.

Kayc M
12,593 PointsStill doesn't work because Array() doesn't accept native array methods such as push(), so this wouldn't work the way I wanted but thanks anyway.
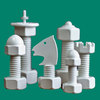
Steven Parker
230,274 PointsThe point of one of my hints is that you shouldn't be using "push" in this case. The elements have been pre-allocated so they only need to have values assigned.
The forEach idea can work if the hints are implemented.
And while not needed here, the "Array" prototype does implement "push". You may have had some other issue. Example:
var test = new Array(2);
test.push("It works!");
console.log(test); // [undefined, undefined, "It works!"]

Kayc M
12,593 PointsYou're right my test on node was
var test = new Array(2);
test[0].push("It works!");
console.log(test); // TypeError: a[0].push is not a function
As if I was accessing an array inside an array
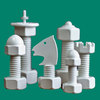
Steven Parker
230,274 PointsAt that point, "test[0]" is still "undefined".

Kayc M
12,593 PointsI don't know what should I reference the num parameter to
createTokens(num) {
const tokens = Array();
tokens.forEach(i => {
let token = new Token(i, this);
tokens.push(token);
});
return tokens;
}
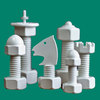
Steven Parker
230,274 PointsThe "num" is the quantity of tokens to create, you had the right idea the first time to use it in constructing the array.
See the example I added to my answer.

Kayc M
12,593 PointsYeah, that's what I wanted but I forgot about the fill method and the original method is far easier, thanks again Steven Parker
Kayc M
12,593 PointsKayc M
12,593 PointsBasically I downloaded the finished project changed the for loop in the Player.js file to a forEach and now it won't work, I did this because I had to test if it would work before I was too deep in the project. Here's the snapshot: https://w.trhou.se/hvj3wtncs5
Steven Parker
230,274 PointsSteven Parker
230,274 PointsNow that I see the original code, here are the issues I notice with the intended replacement:
Steven Parker
230,274 PointsSteven Parker
230,274 PointsApplying the hints would produce something like this:
While this would work, it's not nearly as concise as the original method.