Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial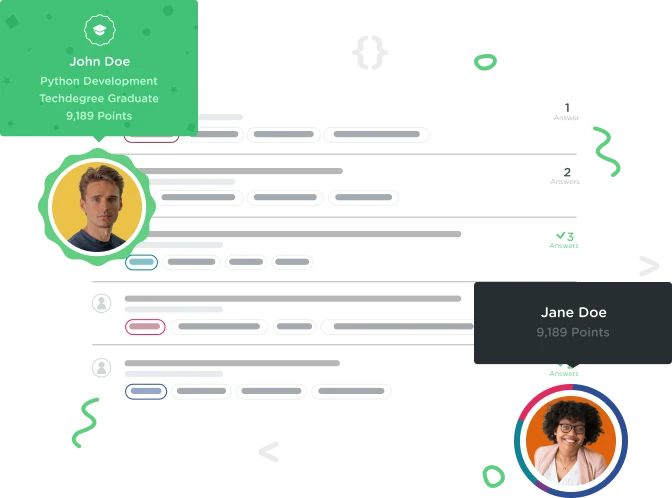

Pranav Mathur
1,061 PointsCan I use a simple JavaScript function (instead of Arrow fn) in a REACT app??
A normal JavaScript fn like, function name(){} instead of () => {}
2 Answers
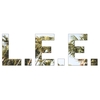
Lee Geertsen
Java Web Development Techdegree Student 16,749 PointsYes you can add a named function to your React class. In the example below I wrote the function add in my React class. You just don't have to put the word function in front of it. But when you want to call the add function, when you click on a button for example, you have to use an arrow function.
class App extends React.Component {
constructor(props) {
super(props);
this.state = { number: 0 };
}
add() {
this.setState(prevState => ({
number: prevState.number+ 1
}));
}
render() {
return (
<div>
Number: {this.state.number}
<button onclick={() => this.add()}>+1</button>
</div>
);
}
}
ReactDOM.render(<App/>, mountNode);
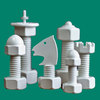
Steven Parker
231,269 PointsThe only concern I might have in replacing an arrow function with a conventional one would be if the code inside the function uses "this". An arrow function doesn't set "this" so it would have the same value as in the context of the definition, but a conventional function establishes a new value for "this".
Steven Parker
231,269 PointsSteven Parker
231,269 PointsWhy would you "have to" use an arrow function? It seems like you could replace the arrow function inside "add" also:
Pranav Mathur
1,061 PointsPranav Mathur
1,061 PointsSo that means, WE CAN use named functions inside components..?!
Lee Geertsen
Java Web Development Techdegree Student 16,749 PointsLee Geertsen
Java Web Development Techdegree Student 16,749 PointsYes you can use named functions inside a component. But if you want that the variable this points to the React component, you'll have to use the arrow functions
Steven Parker
231,269 PointsSteven Parker
231,269 PointsMy example is still anonymous, but it could have been named. And you can also give arrow functions names.