Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial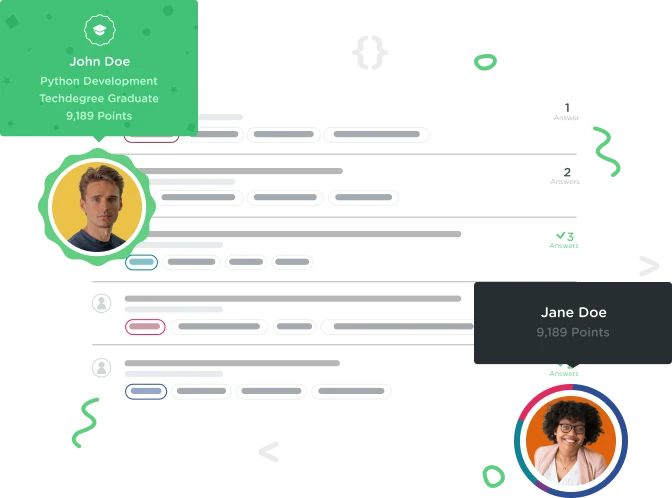
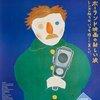
Stanisław Dudzik
9,731 PointsCan i use an argument of a function as a count in {} with regex?
I am having a problem trying to include the argument of my function into regex. I would like to search for a word of a given length(which is an argument of my function find_words) Can I just put an argument in curly brackets like that {count} ? Is that even possible?
import re
def find_words(count, string):
return(re.findall(r'\w{count}', string))
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
6 Answers
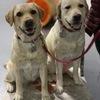
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Stanislaw,
You can achieve what you want but you need to use string concatenation.
Build up your string like this:
pattern = r'some_pattern{' + my_variable + '}'
Cheers
Alex

Gabriel Nunes
6,161 PointsThe other possible way of doing this it using the format method of str. The only issue is that when adding the brackets for the placeholder the brackets for the re parameter must be doubled to be escaped. I attach the code with the answer.
import re
def find_words(count, data):
param = r'\w{{{},}}'.format(count)
return re.findall(param, data)
Other possible way is using the old Python 2 format syntax with the %.
import re
def find_words(count, data):
param = r'\w{%s,}'%(count)
return re.findall(param, data)
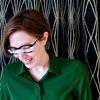
Vanessa Van Gilder
7,034 PointsIf anybody is wondering, this is how you use raw and f-strings together while also having the curly-braces appear:
pattern = fr'\w{{{count},}}'
return re.findall(pattern, text)
You use a double moustache {{ if you actually want it to appear. This does look crazy and string concatenation is probably the easiest to read. But I guess if you're tackling regex...easy reading isn't your priority! https://www.python.org/dev/peps/pep-0498/#escape-sequences
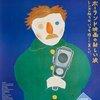
Stanisław Dudzik
9,731 PointsThank you so much for quick response. I am not sure about that solution. I guess it is not possible since my argument is an integral not a string. in my case "must be str, not int" error is raised.
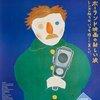
Stanisław Dudzik
9,731 PointsOk, I see. First I need to change my argument into a string like this : str(count) and then concatenate it. Thanks.
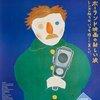
Stanisław Dudzik
9,731 PointsIt worked perfectly fine, but could you think of any other way to go about it?
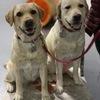
Alex Koumparos
Python Development Techdegree Student 36,887 PointsI've not yet seen a better way.