Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial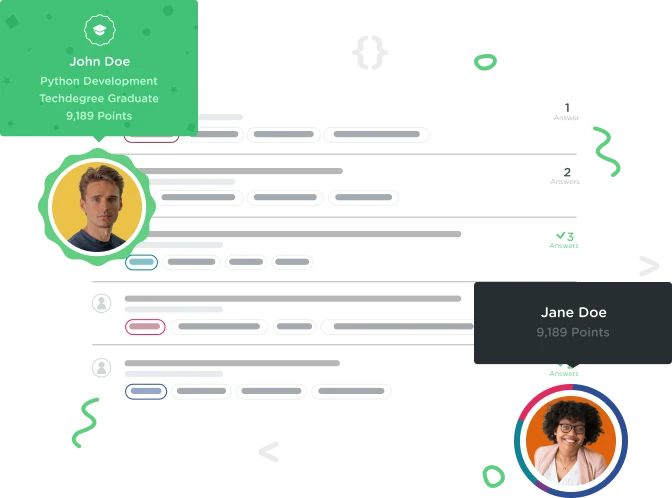

mendelbakaleynik
1,009 PointsCan I use an .index to search for a value that may change?
alpha = "spoons"
beta = "knives"
If I wanted to know the index of the first appearance of any char (let's say "s") from "alpha" in "beta", how would i write that function?
3 Answers
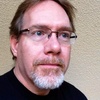
Chris Freeman
Treehouse Moderator 68,423 PointsIf you are set on using index
you could loop over the beta
string:
$ pythonPython 3.4.0 (default, Jun 19 2015, 14:20:21)
[GCC 4.8.2] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> alpha = "spoons"
>>> beta = "knives"
>>> for char in alpha:
... try:
... print(beta.index(char))
... except ValueError:
... print('n/a')
...
5
n/a
n/a
n/a
1
5
>>>
The try
is necessary because index()
raises an error if char
not found.
You could also check if char
is in beta
before indexing, but this is, in effect, searching for the char
twice:
>>> for index, char in enumerate(alpha):
... if char in beta:
... print("alpha char {} (index:{}) found at index {}".format(
... char, index, beta.index(char)))
...
alpha char s (index:0) found at index 5
alpha char n (index:4) found at index 1
alpha char s (index:5) found at index 5

mendelbakaleynik
1,009 PointsThanks for the answers.
But since you were curious about why I needed to use .index, here is the question I am trying to solve.
"Write a function that gets a string named str and a string named set. The function will return the index of the first appearance of any char from set in str.
e.g. - str = "baboonshavelongages2015!", set="t98765!", the function will return 22 (index of '5' in str).
Make sure that time complexity isn't larger than the length of both strings - O(m+n). Assume the string only has ASCII characters."
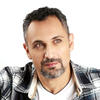
Haydar Al-Rikabi
5,971 PointsAnother way of doing it:
alpha = "spoons"
beta = "knives"
alpha = list(alpha) # convert the string to a list
beta = list(beta)
# This comprehension list is where the main process happens.
# The set() function returns only the unique values in the list (no repeated characters)
mylist = ['The letter {} in alpha is at index {} in beta'.format(x, beta.index(x))
for x in set(alpha) if x in set(beta)]
result = '\n'.join(mylist) # Format the text for neat display
print(result)