Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial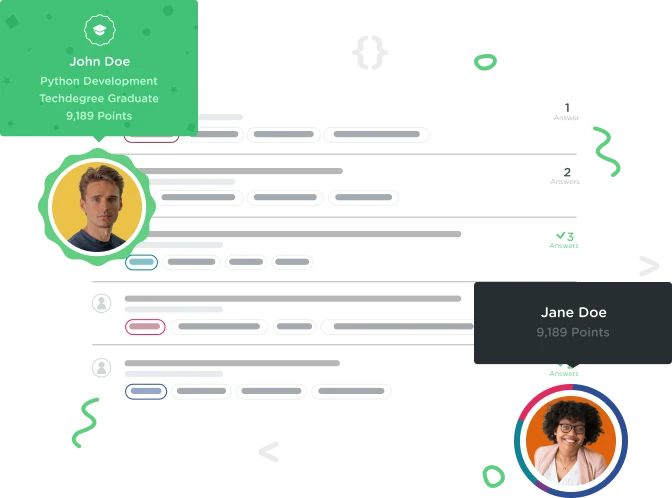

Diego Alvarez
6,680 PointsCan I use global variables within the nested If statement?
I was analyzing the code from this task and I was wondering if the nested if
is always validating the variable that we passed at the main if
?
Can I do it like this, or It would be harder to read / bad practice?
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6 ) + 1;
var guess = prompt('I am thinking of a number between 1 and 6. What is it?');
var guessMore = prompt('Try again. The number I\'m thinking of, is more than ' + guess);
var guessLess = prompt('Try again. The number I\'m thinking of, is less than ' + guess);
if (parseInt(guess) === randomNumber ) {
correctGuess = true;
} else if (parseInt(guess) < randomNumber) {
if (parseInt(guessMore) === randomNumber) {
// var guessMore was here.
correctGuess = true;
}
} else if (parseInt(guess) > randomNumber) {
if (parseInt(guessLess) === randomNumber) {
// var guessLess was here.
correctGuess = true;
}
}
if ( correctGuess ) {
document.write('<p>You guessed the number!</p>');
} else {
document.write('<p>Sorry. The number was ' + randomNumber + '.</p>');
}
Thank you in advance. =)
1 Answer
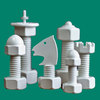
Steven Parker
230,274 PointsGlobal variables are available at any level of nesting as long as they have not been "shadowed" by a variable with the same name in a closer scope.
The "best practice" approach with variables is to declare them in the smallest scope that is adequate for how they are used.
But more than variable scope, the issue here is that the "prompt" statements are no longer controlled by the "if" conditions, so they will both be performed before the values are evaluated. This alters the intended performance of the program.