Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial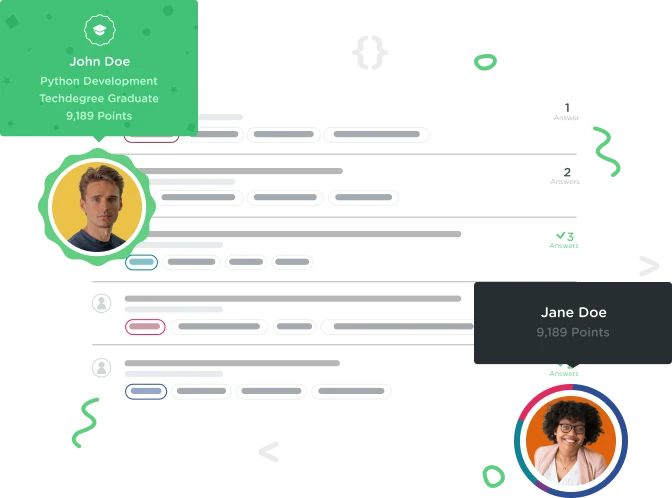
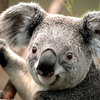
Zach Saul
11,156 PointsCan I use "Typeof" to check if a function argument is an array? (stuck on code challenge)
I am stuck on a code challenge, the task was to create a function which accepts an array and returns it's length but returns 0 if the argument (input) isn't an array. (a string, number, or undefined value)
I wrote:
function arrayCounter (myArray) {
return myArray.length
if (typeof myArray === 'string') { return 0; }
if (typeof myArray === 'undefined') { return 0 }
if (typeof myArray === 'number') return 0 }
}
i want to understand what I'm doing wrong, and whre my error in logic is.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter (myArray) {
return 0;
if (typeof myArray === 'array') {
return myArray.length;
}
</script>
</body>
</html>
2 Answers
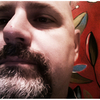
Jeremy Faith
Courses Plus Student 56,696 PointsFirst, you have a return statement as your first line. This will cause the function to return that value and exit out. The other lines of code will not be executed. The typeof used on an array object will return object not array, so probably not what you are looking for. I used Array.isArray(param) and it worked for me. You only have one test and not three.
function arrayCounter(param) {
if ( Array.isArray(param)) {
return param.length;
}else {
return 0;
}
}
Hope this helps.

Chris Shaw
26,676 PointsHi Zach,
See the below link which shows a few different ways to approach this problem from a more intermediate level.
https://teamtreehouse.com/forum/stuck-on-stage-5-functions-quiz