Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial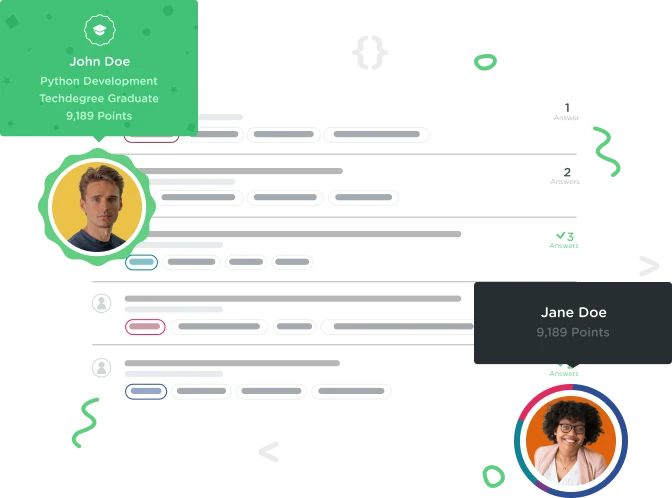

Michael Nanni
7,358 PointsCan instances.py work this way?
I want to solve this challenge using only a single list, and then the combiner function iterates over each item in the list, doing what's needed. I'm getting syntax errors in workspaces in my conditional statement.
The the output from workspaces:
File "combiner.py", line 5
return the_list.join(items) as strings
^
SyntaxError: invalid syntax
Can I even make it work this way? The instructions call for a single list as an argument, but it seems like I might have to set a separate number variable, which I've seen in other solutions.
Any guidance is greatly appreciated!
def combiner(the_list):
the_list = ["pen", 1, "apple", 2, "pineapple", 3]
for item in the_list:
if isinstance(item, str):
return the_list.join(items) as strings
elif isinstance(item, (int, float))
return sum(items) as numbers
result = join(strings, numbers)
print(result)
3 Answers
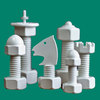
Steven Parker
229,732 PointsHere's a few hints:
- "the_list" is being passed in as an argument, you won't want to assign it to a literal value
- if you "return" inside a loop, the function will end before all items are handled
- you don't need the aliasing operator ("as") in the return statement
- using "join" on a list creates a single string
- the "join" method takes only one argument
- the function needs to "return" the result
- you won't need to "print" anything

Rodney Lyster
2,395 PointsI ended up doing this. I am not sure if it the easiest way, but it worked. I found the length of the list, and looped through adding one until the count was equal to the length. In each loop, i check to see if argument was a str, float or int, and added it to the appropriate variable. Then I returned the string all combined.
It took me a while to figure this one out, and got a lot of help reading the Community Posts.
def combiner(the_list): num = 0 string = "" count = 0 length = len(the_list)
while count < length:
if isinstance(the_list[count], str):
string = string + the_list[count]
count = count + 1
if isinstance(the_list[count], (float, int)):
num = num + the_list[count]
count = count + 1
if count == length:
return "{}{}".format(string, str(num))
print(combiner(["apple", 5.2, "dog", 8]))
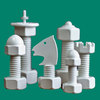
Steven Parker
229,732 PointsOne improvement might be to use "for...in" syntax instead of "while". That would save you from needing to index into the original list or managing a separate "count".
But congratulations on solving it!

Anupam Kumar
3,795 PointsWhat is wrong with this code, I feel this is what being asked
def combiner(strin):
stri=[]
num=0
for i in val:
if isinstance(i, str)==True:
stri.append(i)
if isinstance(i, (int, float))==True:
num+=i
return ''.join(stri)+str(num) ```
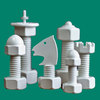
Steven Parker
229,732 PointsYou've posted this as an "answer" to Michael's question, but it's not really an answer and won't be readily seen by other students here.
Instead, start a fresh question and include your code and a link to the course page with it.
Michael Nanni
7,358 PointsMichael Nanni
7,358 PointsHey Steven. Trying to implement your hints here. I removed the return statements in the loop and have only 1 at the end. I'm getting this feedback:
"TypeError: 'int' object is not iterable"
If I'm joining strings and numbers that are contained in a single list, how can I reorder them? (assuming they are not already in order)
Steven Parker
229,732 PointsSteven Parker
229,732 PointsA few more hints:
Michael Nanni
7,358 PointsMichael Nanni
7,358 PointsI really appreciate all your hints Steven, but I don't think I follow this. I'm lacking context here, as the instructions are confusing to me.
I'm trying to follow these instructions as literally as I can, bc it's served me well in past courses. Clearly temporary variables were not something I even considered.
Why do I need temp variables? What is string.append(item) doing in the below solution? How does it contribute to result?
Thanks so much for all your attention.
Steven Parker
229,732 PointsSteven Parker
229,732 PointsIn that solution, the temporary variables "number" and "string" are being used to accumulate the list elements. The "append" method adds an item to the "string" list which is later joined into the final value. Similarly, "number" provides an accumulator for summing the numeric values.