Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial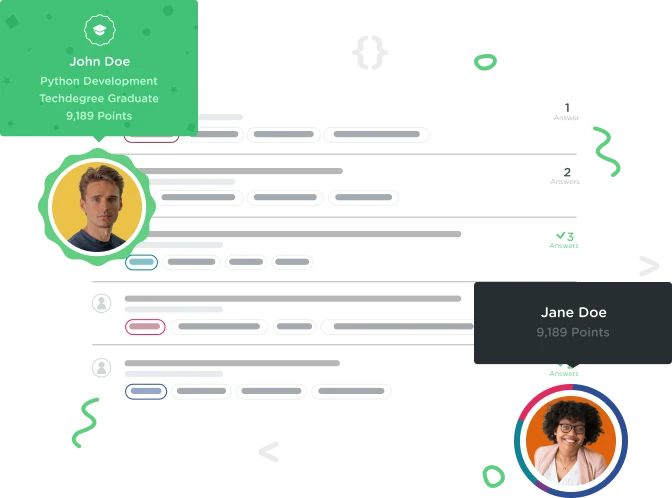
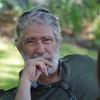
Doug Hawkinson
Full Stack JavaScript Techdegree Student 25,073 PointsCan NetBeans 8.0 be used to perform the exercises in the Java Track? I tried the first exercise. No go.
I tried using NetBeans 8.0 to follow along with the Java Track. I entered the exercise using NetBeans. It would not compile. I then entered the exercise in Workspaces and it compiled and ran fine. That leads me to believe that Workspaces is doing something different behind the scenes than Netbeans is. If I can't use NetBeans that is OK. I just want to know before I waste a lot of time trying.
I am including the code and the compile error just in case it is something simple I can do to NetBeans to make it compatible.
/*
* Treehouse.com - Java Basics
*
* This is the INTRODUCTIONS program
*/
package introductions;
import java.io.Console;
/**
*
* @author westcoasthawk
*/
public class Introductions {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Console console = System.console();
console.printf("Hello, my name is Doug");
}
}
compile error
run: Exception in thread "main" java.lang.NullPointerException at introductions.Introductions.main(Introductions.java:19) /Users/westcoasthawk/Library/Caches/NetBeans/8.1/executor-snippets/run.xml:53: Java returned: 1 BUILD FAILED (total time: 0 seconds)
3 Answers

Seth Kroger
56,414 PointsIDEs like NetBeans have issues with java.io.Console when you use their built-in console. You need to use System.in and System.out instead. System.out works virtually the same as Console for output. You just replace console
with System.out
. When you get to input you need to open a BufferedReader first:
import java.io.BufferedReader;
import java.io.InputStreamReader;
// ...
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
System.out.printf("Make a guess: ");
String guess = bufferedReader.readLine();
Also, Console should work if you use a separate command console. It's just the ones inside the IDE that have the issue.
https://teamtreehouse.com/community/java-ide-question has more info.
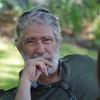
Doug Hawkinson
Full Stack JavaScript Techdegree Student 25,073 PointsSeth: Thanks for the input. I was wondering about that. I have taken one other Java course where we used NetBeans and, as you say, we used System.in and System.out. I appreciate you taking the time to answer.
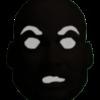
Geovanie Alvarez
21,500 PointsYou can also use Scanner for the input
import java.util.Scanner;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Make a guess: ");
String guess = sc.nextLine();
System.out.println(guess);
}
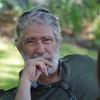
Doug Hawkinson
Full Stack JavaScript Techdegree Student 25,073 PointsAlso a very good answer.
David Spell
899 PointsDavid Spell
899 PointsSeth how would you code "console.readLine("What's your Name") in NetBeans and have it work the same System.in?
Seth Kroger
56,414 PointsSeth Kroger
56,414 PointsSince console.readLine(String) prints out the string you pass in as a prompt before accepting input. It's the same as printing the string out with printf before calling readLine without an argument, just with less code. Without console you you use: