Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial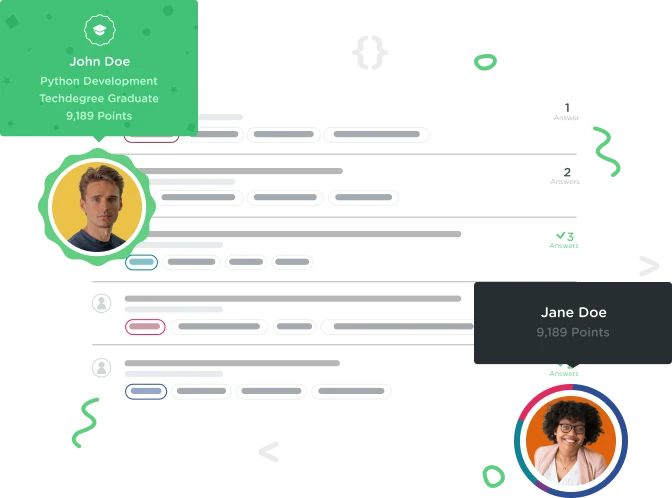

edmond habimana
Courses Plus Student 8,352 PointsCan some check my code. I want to know what i did wrong.
function print(message) {
document.write(message);
}
var quiz = [
["how many states are in the USA?", 50],
["what is 2 + 2 = ?", 4],
["what is 6 + 6= ?", 12],
["what is 7 + 7= ?", 14],
["what is 4 + 4= ?", 8]
]
var answerCorrect = 0;
var questionCorrect;
var questionWrong;
var questions;
for(var i=0; i <= quiz.length; i++){
questionCorrect = "<h2> quetions you got right.</h2><br/>";
questionWrong = "<h2> quetions you got wrong.</h2><br/>";
questions = prompt(quiz[i][0]);
var answers = parseInt(questions);
if (answers === quiz[i][1]){
answerCorrect += 1;
questionCorrect += "<p>" +quiz[i][0]+ "</p><br/>";
}else{
questionWrong += "<p>" +quiz[i][0]+ "</p><br/>";
}
}
print("this is how many quetions you got right: "+answerCorrect);
print(questionCorrect);
print(questionWrong);
2 Answers

Erik Nuber
20,629 PointsOne of the issues you are having is that you reset questionsCorrect and questionsWrong every time you go thru the for loop.
for(var i=0; i <= quiz.length; i++){
questionCorrect = "<h2> quetions you got right.</h2><br/>";
questionWrong = "<h2> quetions you got wrong.</h2><br/>";
questions = prompt(quiz[i][0]);
var answers = parseInt(questions);
if (answers === quiz[i][1]){
answerCorrect += 1;
questionCorrect += "<p>" +quiz[i][0]+ "</p><br/>";
}else{
questionWrong += "<p>" +quiz[i][0]+ "</p><br/>";
}
}
With the way this loop is set up, each time the variable i is incremented and the loop is run through, questionsCorrect and questionsWrong are set to "<h2> quetions you got right.</h2><br/>"; and "<h2> quetions you got wrong.</h2><br/>";.
so moving them out like this will keep them from resetting.
var answerCorrect = 0;
var questionCorrect = "<h2> questions you got right.</h2><br/>";
var questionWrong = "<h2> questions you got wrong.</h2><br/>";
var questions;
var answers;
for(var i=0; i <= quiz.length; i++){
questions = prompt(quiz[i][0]);
answers = parseInt(questions);
if (answers === quiz[i][1]){
answerCorrect += 1;
questionCorrect += "<p>" +quiz[i][0]+ "</p><br/>";
}else{
questionWrong += "<p>" +quiz[i][0]+ "</p><br/>";
}
}
next, in the for loop, you are giving a comparison operator that is pulling an undefined.
i <= quiz.length
this will mean that the final time the loop runs, you aren't actually giving the questions anything
i < quiz.length
will fix the issue as remember that the index value starts at 0 and goes up to one less than the length of the array.
you could also fix this by doing
i <= (quiz.length -1)
which is the same thing.

edmond habimana
Courses Plus Student 8,352 PointsThank a lot Erik, i really appreciate you taking your time checking my code and explaining what i did wrong. I am new at javascript and these silly mistakes were starting to drive me crazy.

Erik Nuber
20,629 PointsIt's not silly mistakes. You are more than fine. It is apart of the learning process. You will find mistakes constantly when you are coding and, it is learning how to find them that will benefit you the most. So no need to apologize or explain. Best of luck as you continue the course.