Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial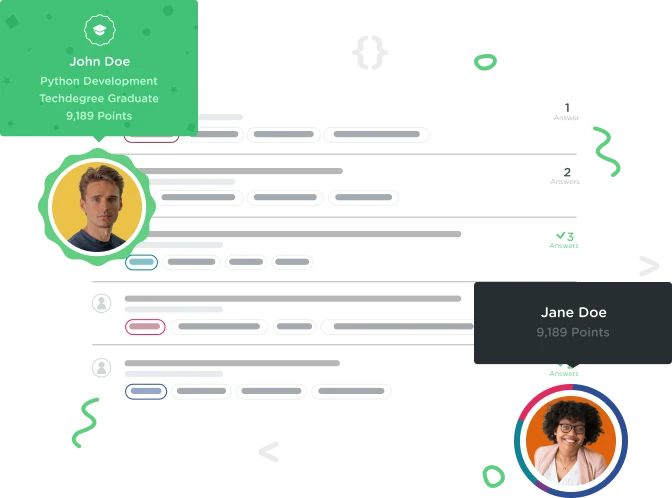

Roy Reuveni
2,621 PointsCan some one elaborate on init functions in sub classes and super classes?
Actually i'm wondering why do we need to have the "super.init" funcion inside the brackets of the "override init". In a logical manner, couldn't we also use:
override init() {} super.init() {}
I also would really appreciate an explanation for: override init(x: Int, y: Int) { super.init(x: x, y: y) }
Why we're using "x: x" and "y: y" in the super.init function, in comparison to the override init?
Thanks :-)!
2 Answers

Stone Preston
42,016 PointsYou call the super class init method inside the subclass override because init methods are designed to set up the object for use. By calling the super class init method in addition to adding your own initialization steps for the subclass, you ensure that the object is set up correctly.
If you didnt call the super class init method inside the subclass init, you may miss out on important initialization steps that the super class method performs.
override init() {}
super.init() {}
is not correct because whenever we create an object of the subclass, it would only call the subclass init method, but the super class method would not be called since its outside the method, thus the object might not be setup correctly.
By placing the call to the super class method INSIDE the subclass method, we ensure that the object is set up correctly and that ALL setup is done.
For example, lets say we had a class called Shape and a subclass of shape called square. The shape class has a numberOfSides property and a color property. In the shape intializer we set the color property to a default of blue.
The square class is just a special kind of shape. In the square class initializer, we want to set the numberOfSides to 4, but we also want to make sure it gets set up correctly as a Shape (squares superclass) AND as a square. So we call the superclass init inside the square class init to make sure its set up as a Shape and a Square. It gets its number of sides set but it also gets its color set (because we call the super class init method inside the subclass init)
If we didnt call the super method, the color would not be set. See why its important to call the super class method inside the subclass? It ensures that the subclass gets initialized correctly. Since a square IS A shape, we want to make sure it gets set up as a Shape first (by calling the superclass init inside the subclass init) , and then set it up as a more specialized type of shape called a square by adding code to the subclass init method
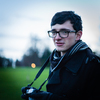
Matthew Spear
14,534 PointsDo you have an example or code you are trying?
It sounds like you don't need to override the initialiser and could be left out. Otherwise it might be a special case and would need more information about your questions.
An example of an inherited initializers:
//: Playground - noun: a place where people can play
import UIKit
var str = "Hello, playground"
class Person
{
var firstName: String
var secondName: String
init(firstName: String, secondName: String)
{
self.firstName = firstName
self.secondName = secondName
}
}
class Spy: Person
{
}
Person(firstName: "Matt", secondName: "Spear")
Spy(firstName: "James", secondName: "Bond")
Give it a try and let me know if you have any other questions!
Matt

Roy Reuveni
2,621 PointsYeah I was asking for the specific case when you create a subclass that uses its superclass definitions. In that case where you should do override the first init. Thanks for the example though Matt!
Roy Reuveni
2,621 PointsRoy Reuveni
2,621 PointsGot it! Thanks Stone! The first part of your answer has made things clear for me as for why we call it inside the subclass method. So as you say if we won't put it inside it won't read and get execute. Also the extra info is definitely adding to my understanding of the relation ship between the two, thank you! important stuff! glad I asked