Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial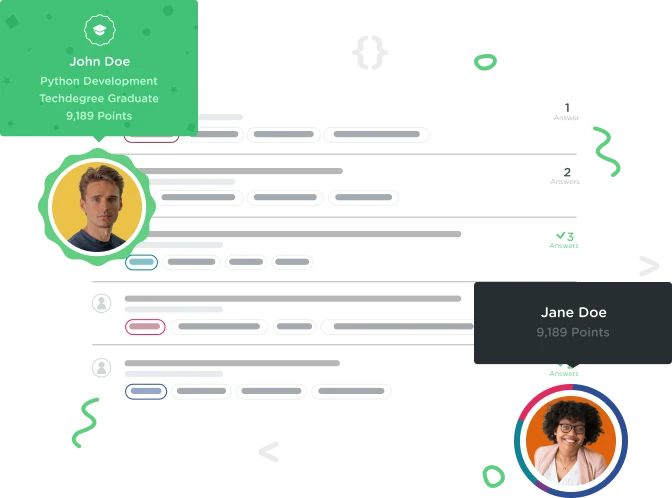

Kendall Kelly
927 Pointscan some one explain While true: continue and break in list to me?
can some one explain While true: continue and break in list to me? i dont get it at all, i dont understand what While true: means or does
1 Answer
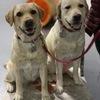
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Kendall,
In most programming languages we have two loop constructs to repeat a block of code. Typically, these are called 'for' loops and 'while' loops. The ideal use case for a for loop is to to repeat something a definite number of times (e.g., repeat some process once for every item in a list, or repeat something 10 times). In contrast, the ideal use case for a while loop is to repeat something while some condition remains true, or until some event happens. For example, searching for a value in a list: you might find it right at the beginning of the list and only go through the loop once, it might be near the end and you have to repeat a bunch of times, or it might not be in the list at all.
When we set up a while loop we have some condition that is true before the loop starts. Inside the body of the loop, we do whatever we want to do and that might change the condition. If it doesn't change the condition, the loop continues as before. If it does change the condition, the loop ends.
Here is a simple example:
hungry = True
while hungry:
food_eaten = eat_food()
if food_eaten == full_meal:
hungry == False
In the above example, we start the loop because we are hungry. Unless we eat a full_meal, we'll stay hungry and the loop will continue forever. As soon as we do eat a full_meal, hungry becomes False and the loop ends.
Loops have a couple of special keywords that let us manipulate the flow of a loop: continue
and break
.
continue
ends the current iteration of a loop but goes back to the beginning of the loop. Let's modify our earlier example to illustrate:
hungry = True
while hungry:
food_eaten = eat_food()
if food_eaten == snack:
continue
if food_eaten == full_meal:
hungry == False
In this example, if we eat a snack, the continue
keyword will trigger, and the current cycle of the loop will end (the computer won't bother checking if a full_meal was eaten, it'll just go straight back to the top of the loop).
We can also use break
to interrupt the flow of the loop, but in this case, as the name suggests, it will end the loop completely:
hungry = True
while hungry:
if starved_to_death:
break
if food_eaten == snack:
continue
if food_eaten == full_meal:
hungry == False
Now, if we've starved to death, there's no point doing any more of the loop at all, we might as well just get out. Using break
gets out the loop immediately and finally. Note if we had just set hungry to False there, we'd still have gone through the whole of the rest of the loop's cycle before ending.
Because we can always use break to escape from a loop, sometimes we deliberately make an infinite loop (a loop with no condition that is ever false) and use break as the way to end that loop.
Here's an example:
while True:
print("This is an infinite loop")
This block will execute forever, because True will never be False.
Here we'll add a break:
while True:
print("This is an infinite loop")
do_something()
if bored:
break
Now, you might rightly be thinking, "Why don't you just make bored the condition?", wouldn't that be the same, and we wouldn't need break?
Yes, we totally could. Just like this:
while not bored:
print("This is an infinite loop")
do_something()
And in this example, the behaviour is basically the same. But consider this slight change:
while True:
print("This is an infinite loop")
do_something()
if bored:
break
do_something_else()
versus this:
while not bored:
print("This is an infinite loop")
do_something()
do_something_else()
Imagine that do_something_else() is a function that takes a really long time, or uses a lot of resources. If do_something() sets bored to True, we have to wait for do_something_else() to finish before we get out of our loop. Using break, we get out of it immediately.
Hope that clears things up.
Cheers,
Alex