Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial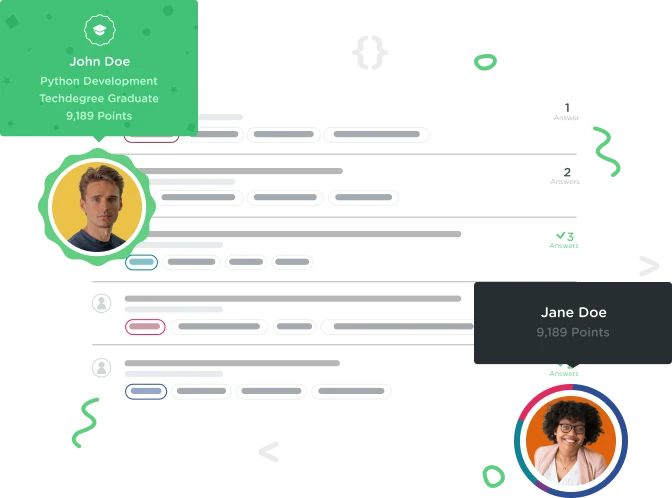

Mostafa Kateb Nejad
927 PointsCan some one help me with constructors, I am having a difficult time understanding how Constructors work ,
can you please look at the question below and advice on how to create a constructor and maybe explain constructors in your own words .
thanks
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
}
}
2 Answers
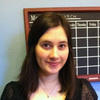
Amber Camus
3,358 PointsIn the last challenge you created a public constructor in GoKart.java. and you said you wanted a String parameter called color to be passed in. You then stored that color in the GoKart constructor as the private color field. Now mColor will match whatever color you pass in when you create a new GoKart in the next step. This step sets everything up to create the GoKart object which is what you're now doing in the Example.java file. Here's what the code looked like for that step which you already completed:
public GoKart(String color){
mColor = color;
}
Here's the next step in Example.java:
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart goKart = new GoKart("purple");
}
}
So, I've created a new GoKart called goKart and I set the color to be purple.
I'm still trying to wrap my mind around constructors so I'm not sure if I explained this well.

La Gracchiatrice
4,615 PointsThe question asks to: 1) create a variable of type GoKart; 2) create a GoKart object using the constructor GoKart(String color); 3) assing the reference of the object 2) to the variable 1).
The constructor creates: a referece to the object, a space in memory for the object and initializes its fields. See https://docs.oracle.com/javase/tutorial/java/javaOO/objectcreation.html
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsIn my own words,
when you instantiate a class you are using a constructor to pass in arguments, if any, for your methods of that class to operate. For example, If you create a class named Dog and you have variables that require input from the user such as String dogName; or int dogHeight; you would create a constructor to accept user input that would then go into your program. So after creating class Dog and I write another class that would instantiate class Dog and input the respective information. Here is a tid bit of code of the initial class and then the class that would instantiate it using user input:
public class Dog { private String name; private int height;
public Dog(String dogName, int dogHeight) { // This is a constructor. There can be multiple constructors as long as //..the argurments are not the exact type and order name = dogName; // this is where user input gets passed from the constructor into the class. height = dogHeight; // this is where user input gets passed from the constructor into the class. } public void someMethod(){ System.out.println("Your dog's name is " + name + " and it is " + height + " inches tall."); } // end method someMethod
} // end class Dog
The class that would instantiate it would look like this passing those arguments:
public class DogInstance{
public static void main(String[] args){ // method main is necessary to run your program. Dog dog = new Dog(Spike, 31); // this instantiates your first class passing two arguments dog.someMethod(); //this calls the method someMethod() which outputs the dog's name and height. } // end method main } // end class DogInstance
The output of your program would be: Your dog's name is Spike and it is 31 inches tall.
I hope this helps.