Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial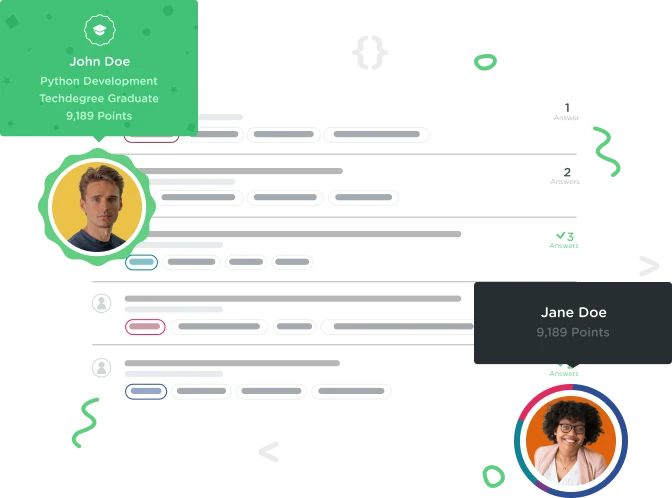

Jason Oliverson
4,230 PointsCan somebody check my code and see where I'm going wrong? I've tried multiple different ways and can't seem to get it
Like I said, I've tried many different ways and checked the other responses but none of them seem to work. I'm starting to think that there's something wrong with the code challenge.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!") /*I deleted the apostrophe in Im (I'm), and my code compiled... Don't know if anyone had this issue, but it wouldn't let me complete the challenge until I made this change. */
}
}
// Enter your code below
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch(direction) {
case "Up":
self.location.y++
case "Down":
self.location.y--
case "Left":
self.location.x--
case "Right":
self.location.x++
default:
break
}
}
}
1 Answer
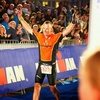
Steve Hunter
57,712 PointsHi Jason,
Firstly, the increment and decrement unary operators have been removed in Swift 3; you can't use ++
or --
any more. Try using += 1
or -= 1
instead. You then need an underscore in the func
as in the method you are overriding. The rest looks fine.
Let me know how you get on.
Steve.
P.S. The apostrophe in the Machine
class is annoying, I agree. You can escape it by preceding the character with a backslash. That will then let your code format work properly.