Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial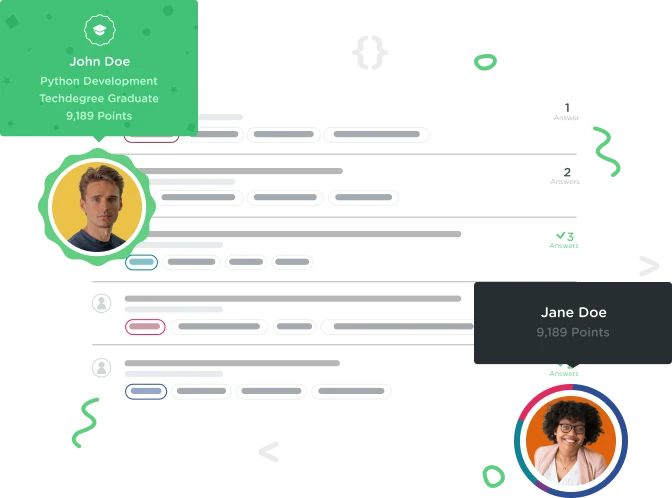
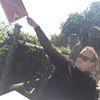
Dennis Klarenbeek
17,168 PointsCan somebody help me to get this challenge done?
Complete the implementation of the merge method in utilities.js file. You should be able to pass in a string with placeholders with percent signs (%) surrounding them. The second parameter should be an object with values to be inserted in to the placeholders. Look at index.js to see how it should work.
var utilities = require("./utilities");
var mailValues = {};
mailValues.first_name = "Janet";
var emailTemplate = "Hi %first_name%! Thanks for completing this code challenge :)";
var mergedContent = utilities.merge(emailTemplate, mailValues);
//mergedContent === "Hi Janet! Thanks for completing this code challenge :)";
function merge(content, values) {
content = content.replace('%first_name%', values.first_name);
return content;
}
module.exports.merge = merge;
3 Answers
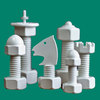
Steven Parker
231,269 PointsYou don't want to merge in just one literal replacement field, you want to merge in any number of fields, based on the values object provided. So you need to loop through the keys from that object and replace things inside the content that match.
If you re-watch the video, you'll see something very similar done but using a different token to surround the keys.
Bryan's approach is an interesting alternative to the method shown in the video, and while it might pass this specific challenge, it's not a general-purpose solution since it makes only one substitution. It could be made more general, but it would require significantly more code than the method shown in the video.
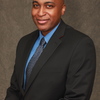
Bryan Knight
34,215 Pointsfunction merge(content, values) {
//first split string based on "%" symbol
var prop = content.split('%');
//split method returns an array so you'll end up with 3 parts
//the part before the first % the part between both % and the part after the last %
//you're concerned with the part between both %. since arrays are zero indexed
//the first part is 0 the part between both % is 1 and part after the last % is 2
// you use the part between both % to access that property name on the values object
//use bracket notation instead of dot notation so that you can use a variable for the property name
//this will replace the placeholder with the actual value found for that property name on the values object
prop[1] = values[prop[1]];
//now rejoin the string and store the result in content
content = prop.join('');
//now return the result
return content;
}
let me know if anything doesn't make sense.
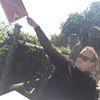
Dennis Klarenbeek
17,168 PointsThank Bryan and Steven,
Both methods works for me but indeed the solution of Steven makes this more general.