Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial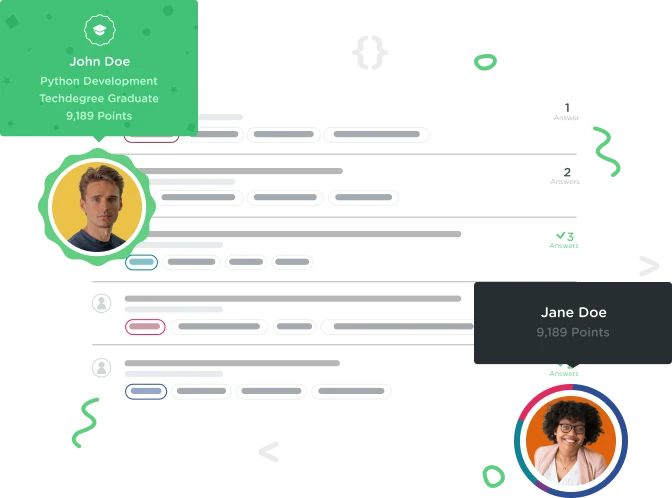
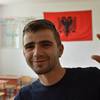
Angjelin Malaj
5,983 PointsCan somebody Help me with this assignment because I am new to java and I would like to know an example of this solution!
Write a programs that given an arrays of coin flips list and count the number of heads or tails as follow:
Input:
coinFlips = { "Tail", "Tail", "Head", "Tail", "Tail", "Tail", "Head", "Head", "Tail", "Head" };
Output:
Heads
index 2
index 6
index 7
index 9
Total heads: 4
Tails
index 0
index 1
index 3
index 4
index 5
index 8
Total tails: 6
Finally,
Add a method that randomly generates and return the array coinFlips
1 Answer
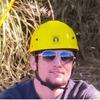
Filipe S Jonson
10,381 Pointspackage questions;
import java.util.Random;
/**
* Created by lispa on 02/05/2016.
*/
public class Teamtreehouse {
public static void main(String[] args) {
String[] coinFlips = coinFlipGenerator(); //calling the method coinFlipGenerator in order to generate the string
System.out.printf("Heads:\n"); // printing the word Heads
result("Head",coinFlips); // calling the method and passing the variables needed , Head and the array
System.out.printf("Tail:\n"); //printing the word Heads
result("Tail",coinFlips); //calling the method and passing the variables needed , Tail and the array
}
public static void result(String word, String[] coinFlips) {
int count = 0; // creating ta a variable to count the numbers of heads or tails
for (int i = 0; i < coinFlips.length; i++) { // for looping trough the array
if (coinFlips[i] == word){ // verifies if the array index contains the word
count += 1; // if it contains add the variable count + 1 "count = count + 1;"
System.out.printf(count + ".index " + i + "\n"); // print in the screen (the actual count + i = the array index)
}
}
System.out.printf("Total " + word + "s: " + count + "\n"); // print the count
}
public static String[] coinFlipGenerator(){
String[] coinFlips = new String[9]; // declare the array
Random randomGenerator = new Random(); // declare a random variable for future use
for (int i = 0; i < coinFlips.length; i++) {
int zeroOrOne = randomGenerator.nextInt(2); // the randomGenerator.nextInt(2) is going to generate a number between 0 and 1
if(zeroOrOne == 0){ // if it's 0 the array index will receive Head
coinFlips[i] = "Head";
}else {
coinFlips[i] = "Tail"; // if it's 1 the array index will receive Tail
}
}
return coinFlips; // return the random array
}
}
Angjelin Malaj
5,983 PointsAngjelin Malaj
5,983 PointsThank you so much Filipe