Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial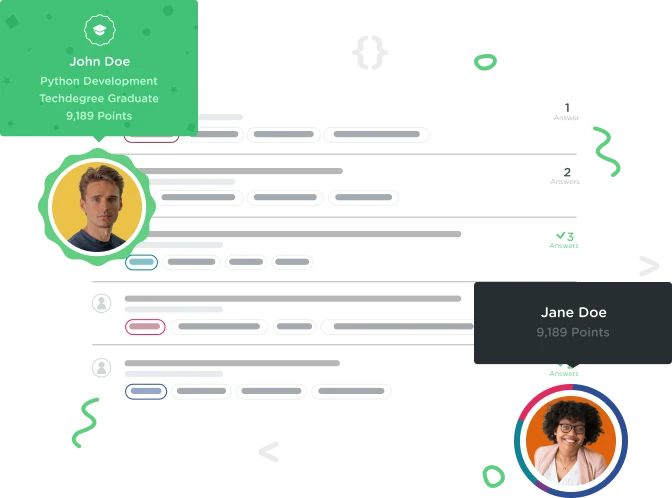

angel Melendez
Courses Plus Student 457 Pointscan somebody tell me whats wrong with my code?
public class Example {
public static void main(String[] args) { // Your amazing code goes here... System.out.println("we are making a new Pez Dispenser"); PezDispenser dispenser = new PezDispenser("Rafael"); System.out.printf("The dispenseris %s ", dispenser.getcharacterName() ); }
} class PezDispenser { Private String characterName;
public PezDispenser(String name0{ characterName= name; }
public String getCharacterName(){ return characterName; }
1 Answer
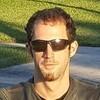
Joshua Kaufman
19,193 PointsFor your Example.java file...
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new PEZ Dispenser");
PezDispenser dispenser = new PezDispenser("Rafael");
System.out.printf("The dispenser is %s", dispenser.getCharacterName());
}
}
Although you don't technically NEED to include %n in the line containing System.out.println("We are making a new PEZ Dispenser");, it is good practice to include it for ease of reading. You can also break up some of the code using enter/return key as you wish.
This is what your PezDispenser.java file should look like
class PezDispenser {
private String characterName;
public PezDispenser(String characterName){//PezDispenser Constructor
this.characterName = characterName;
}
public String getCharacterName(){
return characterName;
}
}
Instead, you had a random "0" character in your constructor function (The second one in the file) instead of properly putting a closing parenthesis ")" ---- Syntax Error.
Error 2 in your class definition is that you used "Private" instead of "private" to define String characterName. This word is CASE SENSITIVE, so be especially careful with that!
In conclusion, your errors appear to have been in your PezDispenser.java file, and those issues were a matter of syntax. Try copying and pasting the code from my markdown and see if it runs. If not, let me know and I'll look at it again.
Gabe Olesen
1,606 PointsGabe Olesen
1,606 PointsHey,
Can you edit your code and put the markdown so it's easier to read?
Markdown sheet is available under "Edit" then at the bottom of the box it will say "Reference this Markdown Cheatsheet for syntax examples for formatting your post."
I'll be glad to help! Get back to me!
~Gabe