Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial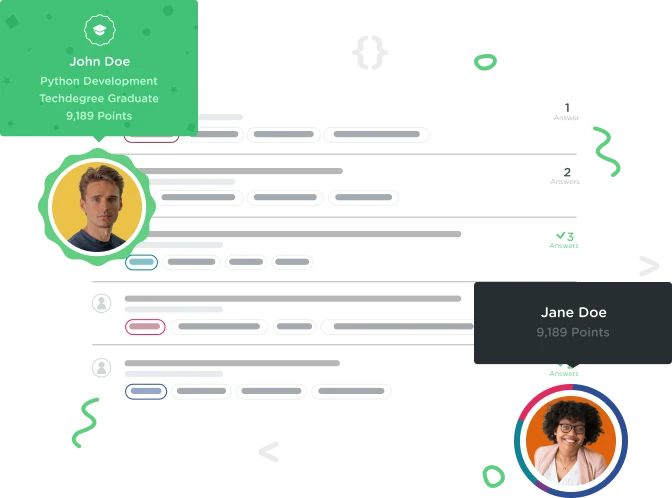

Tsvetan Rankov
Courses Plus Student 385 PointsCan somebody upload the full JavaScript code for this project, please?
I have some problems with my code and some things do not work as expected.
1 Answer

Jeff Xu
10,291 Pointscomplete code plus do-it-yourself tasks at the end of the vid
//Problem: user interaction doesnt provide desired results
//solution: add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById('incomplete-tasks'); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//create a li from new task input
var createNewTaskElement = function(taskString) {
//create list item
var listItem = document.createElement('li');
//input checkbox
var checkBox = document.createElement('input');
//label
var label = document.createElement('label');
//input (text)
var editInput = document.createElement('input');
//button.edit
var editButton = document.createElement('button');
//button.delete
var deleteButton = document.createElement('button');
//each element needs modifing
checkBox.type = "checkBox";
label.innerHTML = taskString;
editInput.type = "text";
editButton.className = "edit";
editButton.innerHTML = "Edit";
deleteButton.className = "delete";
deleteButton.innerHTML = "Delete";
//each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//add a new task
var addTask = function() {
if(taskInput.value == "") {
}
else {
console.log("Add task...");
//create a new list item with text from #new-task
var listItem = createNewTaskElement(taskInput.value);
//append listItem to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
// var checkBox = listItem.querySelector("input[type='checkbox']");
// checkBox.onchange = taskCompleted;
bindTaskEvents(listItem,taskCompleted);
taskInput.value = "";
}
}
//edit an existing task
var editTask = function() {
console.log("Edit task...");
//toggle parent edit mode
var listItem = this.parentNode;
var label = listItem.querySelector('label');
var editInput = listItem.querySelector('input[type="text"]');
var containsClass = listItem.classList.contains('editMode');
var editButton = listItem.querySelector('button.edit');
if(containsClass) {
label.innerHTML = editInput.value;
editButton.innerHTML = "Edit";
}
else {
editInput.value = label.innerHTML;
editButton.innerHTML = "Save";
}
listItem.classList.toggle('editMode');
}
//delete an exisiting task
var deleteTask = function() {
console.log("Delete task...");
//remove li from ul
var listItem = this.parentNode;
var mainList = listItem.parentNode;
mainList.removeChild(listItem);
}
//mark a task as complete
var taskCompleted = function() {
console.log("Task complete...");
//append incompleted task to completed task ul
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem,taskIncomplete);
// var checkBox = listItem.querySelector("input[type='checkbox']");
// checkBox.onchange = taskIncomplete;
}
//mark task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
//append completed task to incomplete task
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem,taskCompleted);
// var checkBox = listItem.querySelector("input[type='checkbox']");
// checkBox.onchange = taskCompleted;
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type='checkbox']");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit taskListItem
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to checkBox
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to addTask
//addButton.onclick = addTask;
addButton.addEventListener('click',addTask);
//cycle over incompleteTasksHolder ul list items
for(var i=0; i<incompleteTasksHolder.children.length; i++) {
//bind events to list items children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i],taskCompleted);
}
//cycle over completedTasksHolder ul list items
for(var i=0;i<completedTasksHolder.children.length;i++) {
//bind events to list items children (taskIncompleted)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
Alice Huang
20,335 PointsAlice Huang
20,335 PointsHi Tsvetan. You can scroll down the page. Below the video there's a menu for Teacher's notes, Question and Downloads. Click Downloads and choose the first option "Project Files".