Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial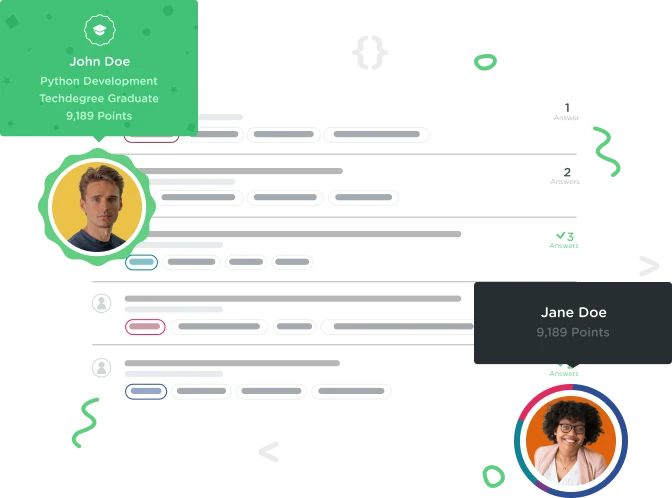
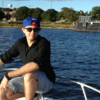
Evan N
5,097 PointsCan someone break down how sort is taking the values, subtracting, and returning them to obtain the correct answer?
If someone can break down each step on how the function is taking each string within the second array. I am still not fully understanding how this is being done.
saying2.sort(function(a, b) {
return a.length - b.length
});
1 Answer

Daniel Reedy
25,284 PointsIt's always good to go to the docs first. The Array.prototype.sort() page is full of useful information, but it doesn't dive as deep as what I think you want to know.
To start with, you'll want to understand what happens with the result of your function call, function(a, b) { ... }
.
The possible return values must fall into one of the following Categories:
Value is less than 0: The lower index item (a
) comes first.
Value is 0: a & b are unchanged in their position to each other, but are sorted compared to other items in the array.
Value is greater than 0: The higher index item (b
) comes first.
Time for an example. Given we have an array of fruit var fruits = ['Apple', 'Banana', 'Orange', 'Pear', 'Tomato']
we want to sort the longest name to the shortest name.
fruits.sort(function(a,b) {
var difference = b.length - a.length;
// If difference is <0 then a is longer than b
// If difference is 0 then they are the same length
// If difference is >0 then b is longer than a
return difference;
}
If you are testing this in the console you can add a line to the method and see what is being compared, console.log(a + " vs " + b + " = " + difference);
Run it and you'd see something like this (with my comments added in):
Apple vs Banana = 1 // Index 0: Banana moves to index 0, Apple to index 1
Apple vs Orange = 1 // Index 1: Orange moves to index 1, Apple to index 2
Banana vs Orange = 0 // Index 0: No change
Apple vs Pear = -1 // Index 2: Apple stays in index 2, Pear to index 3
Pear vs Tomato = 2 // Index 3: Tomato moves to index 3, Pear to Index 4
Apple vs Tomato = 1 // Index 2: Tomato moves to index 2, Apple to index 3
Orange vs Tomato = 0 // Index 2: No change
["Banana", "Orange", "Tomato", "Apple", "Pear"] // Everything compared & our final sorted array
Depending on the browser the underlying sort algorithm used is typically either a merge-sort or quick-sort. If you want to know how those work by a visual, what better way than through Romanian folk dancing? Merge-sort and Quick-sort.
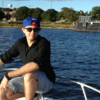
Evan N
5,097 PointsDaniel Reedy Thank you very much for looking into this. This now makes perfect sense, I will also use MDN as a reference point as suggested.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsEvan N ,
You have the option of selecting "Best Answer" if an answer solves your problem.
It gives the answerer some recognition and points and lets others helping in the forums know that this question has been solved.