Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial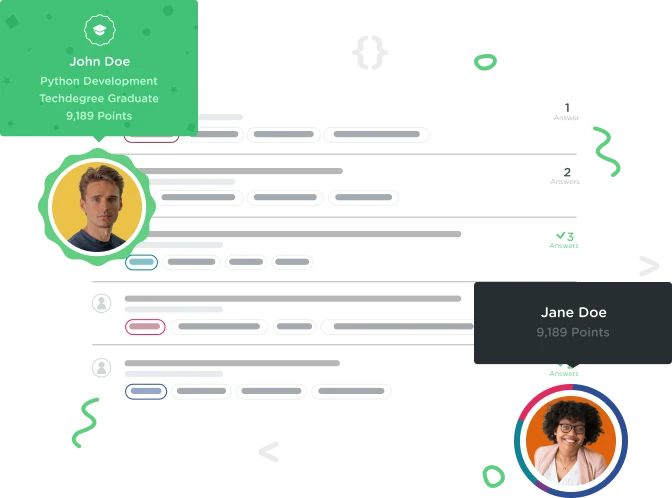
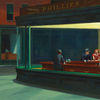
Anthony Grodowski
4,902 PointsCan someone check if there are some not pythonic solutions?
I've done this Quiz file pretty much alone. It works perfectly fine but I want to ask if there are some things I could have done better/in a better style. Also I was wondering if there is a way to enter my logged informations directly and for example append chosen ones to a list.
import datetime
import random
import logging
import os
from questions import Add, Multiply
logging.basicConfig(filename='game.log', level=logging.DEBUG)
class Quiz:
def __init__(self, questions=[], answers=[], score=[], time=[]):
self.questions = questions
self.answers = answers
self.score = score
self.time = time
index = 1
for question in range(10):
self.questions.append("{}".format(random.choice([Add(random.randrange(1, 11), (random.randrange(1, 11))).text, Multiply((random.randrange(1, 11)), (random.randrange(1, 11))).text])))
index += 1
# generate 10 random questions with numbers from 1 to 10 DONE
for question in questions:
question = question.split(' ')
if question[1] == '+':
self.answers.append(Add(int(question[0]), int(question[2])).answer)
elif question[1] == 'x':
self.answers.append(Multiply(int(question[0]), int(question[2])).answer)
def __str__(self):
return str(self.questions)
# add these questions into self.questions DONE
def clear_screen(self):
os.system('cls' if os.name == 'nt' else 'clear')
def ask(self):
index = 0
for question in self.questions:
print(question)
user_answer = input("What's the answer? ")
if int(user_answer) == self.answers[index]:
self.score.append(1)
logging.info(True)
index += 1
else:
self.score.append(0)
logging.info(False)
index += 1
self.clear_screen()
# log the start time DONE
# capture the answer DONE
# check the answer DONE
# log the end time DONE
# if the answers right senf back True DONE
# otherwise send back False DONE
# send back the elapsed time DONE
def take_quiz(self):
start = datetime.datetime.now()
logging.info("Beginning of the quiz: {}".format(start))
self.ask()
end = datetime.datetime.now()
logging.info("The ending of the quiz: {}".format(end))
your_time = end - start
self.your_time = your_time
self.summary()
# log the start time DONE
# ask all of the questions DONE
# log if they got the question right DONE
# log the end time DONE
# show a summary DONE
def summary(self):
print("You got {} out of {} questions right.".format(sum(self.score), len(self.questions)))
print("You finished your quiz in {} seconds.".format(self.your_time.seconds))
print("Here are the lists of the questions and valid answers:\nQuestions: {} \nAnswers: {}".format(self.questions, self.answers))
# print how many u got right and the total of questions. 5/10 DONE
# print the total of time for the quiz. 30 seconds DONE
1 Answer

Kyrylo Troian
570 PointsThis will make static values, data will be saved between calls. Is this an expected behavior?
def __init__(self, questions=[], answers=[], score=[], time=[]):
Too many columns, you can separate them with \, or just moving on the next line.
self.questions.append("{}".format(random.choice([Add(random.randrange(1, 11), (random.randrange(1, 11))).text, Multiply((random.randrange(1, 11)), (random.randrange(1, 11))).text])))
This loop is in the constructor, consider creating a new function and move all of the stuff there.
for question in range(10):
Not very pythonic.
Explicit is better than implicit. Simple is better than complex.
self.answers.append(Add(int(question[0]), int(question[2])).answer) ... return str(self.questions)
Consider using more variables:
name = str(self.questions)
return name
For the next, if you can avoid ternary operators - avoid them.
os.system('cls' if os.name == 'nt' else 'clear')
Also, ask method is called only from inside the class, consider making it private/protected as well as all components that is not meant to be used from outside.
Overall, You are doing great. Never give up!