Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial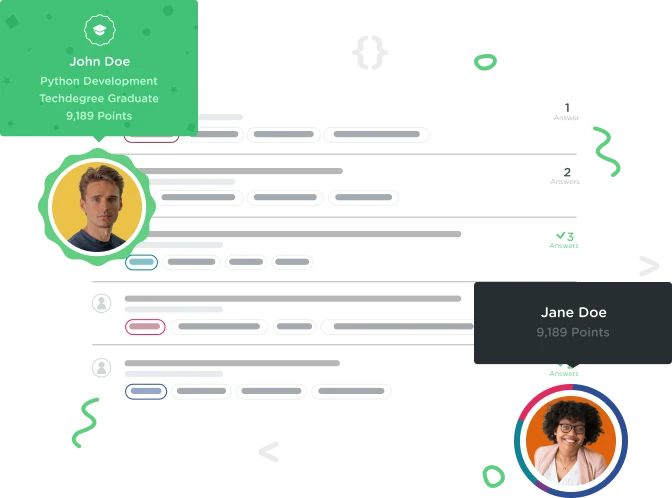
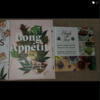
Boss Monkey
4,948 PointsCan someone critique my code?
I have some changes in my version of the quiz challenge.
- I wanted the question counter to be accurate to what question it was in order from 1-5.
- I wanted the answer to be displayed with the question in case the user forgot his answer or wanted to see what the correct answer was on a question he/she got wrong.
// Counts the question answered correctly
var correctQuestions = 0;
//Adds the right/wrong questions to their corresponding array.
var correctQuestionsArray = [];
var wrongQuestionsArray = [];
//2 dimensional array for questions
var questions = [
['Which language reminds you of a gem?', 'ruby'],
['Which language reminds you of a snake?', 'python'],
['Which language was facebook built on?', 'php'],
['Which language reminds you of coffee?', 'java'],
['Which language is used to style?', 'css']
];
//premade function to print on document
function print(message) {
document.write(message);
}
//This for loop will itterate through the questions array
for (var i = 0; i < questions.length; i++) {
//Sets the answer typed by the user to a variable
var answer = prompt(questions[i][0]);
//sets a variable to the question
var questionNumber = i;
//Checks if the user input is equal to the question's answer
if ( answer.toLowerCase() === questions[i][1] ) {
//if equal add 1 to the question number so it starts at 1
questionNumber++;
//add the question number, adds the question, and then adds the answer to variable
correctQuestionsArray += questionNumber + '. ' + questions[i].shift() + ' <b>Answer: </b>' + questions[i].pop() + '<br><br>';
//Adds 1 to the question counter
correctQuestions++;
//if not equal
} else {
//it still adds 1 to the question number (I wanted the question number to be acurate)
questionNumber++;
//add the question number, adds the question, and then adds the answer to variable
wrongQuestionsArray += questionNumber + '. ' + questions[i].shift() + ' <b>Answer: </b>' + questions[i].pop() + '<br><br>';
}
}
//prints a counter of how many questions you got right
print('<p>You got ' + correctQuestions + ' question(s) right.</p>');
//prints the questions you got correct
print('<h4>You got these questions correct:</h4>');
print(correctQuestionsArray);
//prints the questions you got wrong
print('<h4>You got these questions wrong:</h4>');
print(wrongQuestionsArray);
4 Answers

Antti Lylander
9,686 PointsI played with your code a little. My code below still has a problem with printing now that the correct and wrong questions are really stored as an array. Your code actually stored them as a string. Storing in an array let's you use array.length to count correct answers. I also simplified the way you tracked questionNumber.'
Also see how i have changed the way the questions are added on the correctQuestionsString. Your way destroys the original questions array.
// Counts the question answered correctly
//var correctQuestions = 0;
//Adds the right/wrong questions to their corresponding array.
var correctQuestionsArray = [];
var wrongQuestionsArray = [];
//2 dimensional array for questions
var questions = [
['Which language reminds you of a gem?', 'ruby'],
['Which language reminds you of a snake?', 'python'],
['Which language was facebook built on?', 'php'],
['Which language reminds you of coffee?', 'java'],
['Which language is used to style?', 'css']
];
//premade function to print on document
function print(message) {
document.write(message);
}
//This for loop will itterate through the questions array
for (var i = 0; i < questions.length; i++) {
var correctQuestionsString = '';
var wrongQuestionsString = '';
//Sets the answer typed by the user to a variable
var answer = prompt(questions[i][0]);
//sets a variable to the question
var questionNumber = i + 1
//Checks if the user input is equal to the question's answer
if ( answer.toLowerCase() === questions[i][1] ) {
//if equal add 1 to the question number so it starts at 1
//questionNumber++;
//add the question number, adds the question, and then adds the answer to variable
correctQuestionsString += questionNumber + '. ' + questions[i][0] + ' <b>Answer: </b>' + questions[i][1] + '<br><br>';
correctQuestionsArray.push(correctQuestionsString);
//Adds 1 to the question counter
//correctQuestions++;
//if not equal
} else {
//it still adds 1 to the question number (I wanted the question number to be acurate)
//questionNumber++;
//add the question number, adds the question, and then adds the answer to variable
wrongQuestionsString += questionNumber + '. ' + questions[i][0] + ' <b>Answer: </b>' + questions[i][1] + '<br><br>';
wrongQuestionsArray.push(wrongQuestionsString);
}
}
//prints a counter of how many questions you got right
print('<p>You got ' + correctQuestionsArray.length + ' question(s) right.</p>');
//prints the questions you got correct
print('<h4>You got these questions correct:</h4>');
print(correctQuestionsArray);
//prints the questions you got wrong
print('<h4>You got these questions wrong:</h4>');
print(wrongQuestionsArray);
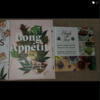
Boss Monkey
4,948 PointsI see, thanks a bunch that really helps!

Victor Adeshile
1,221 PointsIt is a good practice that you put scope into consideration when using the for loop. It is always better to define your variables outside the for loop so that, it is global. Check my code and take note of the difference. Cheers as we all learn and grow our understanding. Cheers.
function qprint(message){
document.write(message);
}
var quest = [
["What is the name of the most popular programming language?"],["JavaScript"],
["What is Victors profession?"],["Programmer"],
["Best color?"], ["White"]
];
// prompt the question and put each question in a prompt function and repeat each question.
var q1;
var q2;
var q3;
var correct = 0;
var wrong = 0;
var corList = [];
var wroList = [];
for(var i = 0; i < quest.length; i+= 6){
q1 = prompt(quest[0][0]);
q2 = prompt(quest[2][0]);
q3 = prompt(quest[4][0]);
}
if(q1 === quest[1][0]){
correct += 1;
corList.push(quest[0][0]);
}else{
wrong += 1;
wroList.push(quest[0][0]);
}
if (q2 === quest[2][0]) {
correct += 1;
corList.push(quest[2][0]);
} else {
wrong += 1;
wroList.push(quest[2][0]);
}
if (q3 === quest[5][0]) {
correct += 1;
corList.push(quest[4][0]);
} else {
wrong += 1;
wroList.push(quest[4][0]);
}
if (correct > 1){
qprint(`You got ${correct} question(s) correctly.`);
}else{
qprint(`You got ${correct} question correctly.`);
}
qprint(`<h1>You got these following question.</h1>`);
function printCorrect(corr){
var lists = '<ol>';
for (var i = 0; i < corr.length; i += 1) {
lists += '<li>' + corr[i] + '</li>';
}
lists += '</ol>';
qprint(lists);
}
printCorrect(corList);
qprint(`<h1>You got these questions wrong.</h1>`);
printCorrect(wroList);

Antti Lylander
9,686 PointsI beg to disagree. It is not a good idea to make all variables global. It's called cluttering the global namespace. Keep all variables private to a function if possible. Make it global only when necessary.

Victor Adeshile
1,221 PointsYes, you said to a function. We are talking about "for loops" here, not functions. Since the loop is only meant to iterate, it is not usually professional to define a variable in a for loop, but you can define it outside, and then call it. Read again my post. Cheers.

Antti Lylander
9,686 PointsYou are right probably. I'm on mobile and your word "global" just jumped at me. Cheers!