Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial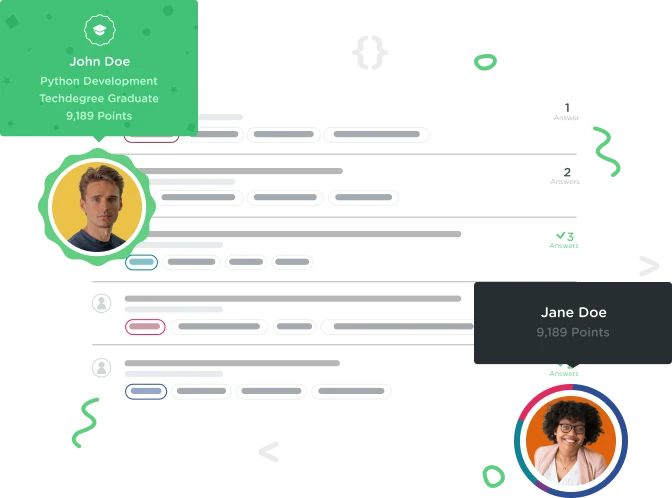

Humberto Hernandez
2,912 PointsCan someone explain how the constructor exactly works here?
My answer was correct when I submitted it, but I would like to know a bit more as to why its correct and how each bit works, thank you!
public class GoKart {
private String mColor = "red";
public GoKart (String color) {
mColor = color;
}
public String getColor() {
return mColor;
}
}
1 Answer
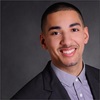
Mario Blokland
19,750 PointsI will try to explain every bit as simple as possible.
public class GoKart {
}
This section declares the class. A class is like a blueprint of an object which you may want to create. Inside the class you define its attributes and how an instance of it (an actual created object) should behave - e.g. like drive, stop, break and so on.
private String mColor = "red"; //declaration + initialization
This line declares a variable named mColor of the type String
. It also initializes the variable with a value, which is the string red
. The mColor variable, is also called an attribute of the class GoKart.
public GoKart (String color) { //declaration
mColor = color; // assignment
}
The constructor always gets called, when you create a new object of the class GoKart. In this case, you would also need to provide a parameter of the type string, in order to create the object because we said so within in parenthesis. The given parameter will then be set as the value of our attribute mColor
.
You would create an instance of the class like this:
GoKart myBeatifulGoKart = new GoKart("purple");
Ok, now the last one:
public String getColor() { //declaration
return mColor; // return statement
}
This here is called an instance method. The signature tells the method, that we expect it to return a value of the type string
. In our case, it would return the string purple
, because we created a car with this color (the current value "red" of mColor will be overwritten).
If you have any questions or something wasn't clear, just let me know.
markus Nowak
581 Pointsmarkus Nowak
581 Pointsnice! thank you very much for the explanation