Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial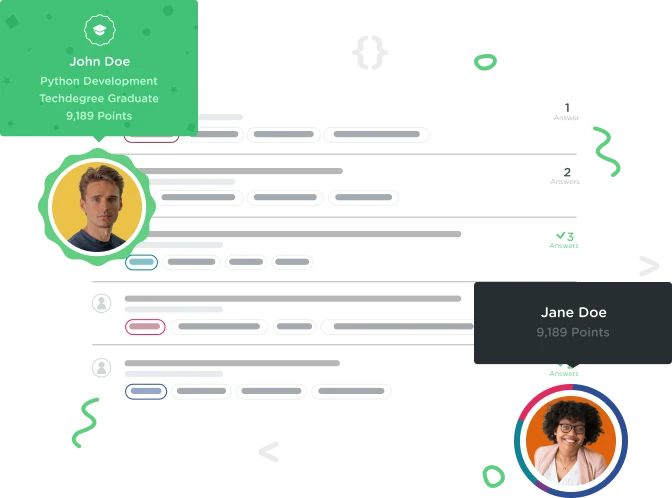

Brian Patrick
Courses Plus Student 2,770 PointsCan someone explain how we're grabbing the PLAYERS array when we use the map function.
I coded everything in the video and it works, I just don't understand how/why.
The array of objects
var PLAYERS = [
{
name: "Brian",
score: 34,
id: 1,
},
{
name: "Frank",
score: 12,
id: 2,
},
{
name: "Joji",
score: 31,
id: 3,
}
];
Player component
function Player(props) {
return (
<div className="player">
<div className="player-name">
{props.name}
</div>
<div className="player-score">
<Counter score={props.score} />
</div>
</div>
);
}
The map function
{props.players.map(function(player) {
return <Player name={player.name} score={player.score} key={player.id} />
Where are we pointing the map function to the PLAYERS array?

Brian Patrick
Courses Plus Student 2,770 PointsHow does PLAYERS become props.players? How are we able to define PLAYERS as props.players and why aren't we using props.PLAYERS.map.
2 Answers

Sipann A.
30,234 PointsHi,
At approx. 4:10, he supplies a "players" property to <Application players={PLAYERS} />
in ReactDOM.render()
call.
So props.players
of Application is assigned the variable PLAYERS
(which happens to be an array of objects).
Hope this is what you're asking
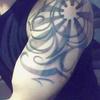
Paolo Scamardella
24,828 PointsThis is because Jim is passing PLAYERS as a prop to the Application component.
// The name of the prop is called players not PLAYERS.
// To use the props inside the Application component, you access it using props.players
// PLAYERS is passed into players props.
ReactDOM.render(<Application players={PLAYERS} />, document.getElementById('container'))
// Why aren't we using props.PLAYERS.map?
// Once again, Application component props is players, not PLAYERS. Jim is just passing PLAYERS to player props.
// If you really want to use props.PLAYERS.map instead of props.players.map,
// you need to change a couple of places:
ReactDOM.render(<Application PLAYERS={PLAYERS} />, document.getElementById('container'))
// In Application return statement
{props.PLAYERS.map(function(player) {
return <Player name={player.name} score={player.score} key={player.id} />
})}
// The important thing to understand about props is you can called it whatever you want it to be.
// For example, you can call it users like:
ReactDOM.render(<Application users={PLAYERS} />, document.getElementById('container'))
// and then inside Application component, to access the array of players, you use props.users like:
{props.users.map(function(player) {
return <Player name={player.name} score={player.score} key={player.id} />
})}
Paolo Scamardella
24,828 PointsPaolo Scamardella
24,828 PointsI'm not understanding your question about the map function. The map function is a standard javascript array method that loops over each element in the array and transform each element in the array depending on the return statement.
Also, it is very important to understand the map function is a functional method that returns a new array and never modifies the original array.
In your case, you are looping over each player in the players array, which is an array of players, and the map function is transforming each player into a react Player component. When looping over, each player inside players array is passing its own information (name, score, and id) to the react Player component.