Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial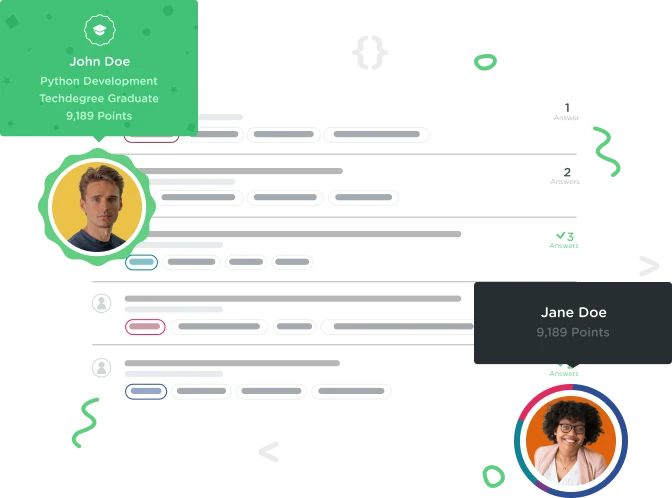
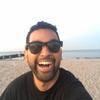
Anthony Albertorio
22,624 PointsCan someone explain tagging and template literals in a simpler way?
The video was pretty vague on how template literals and tagging work. The instructor spent more time explaining how things worked in ES5 rather than in ES6. After reading the Mozilla docs, I understand somewhat how template literals work. However, I feel like the video left out the truly powerful applications of template literals by not explaining how tagging works.
2 Answers
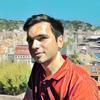
Dan-Lucian Rosu
1,217 Pointsvar person = 'Mike';
var age = 28;
function myTag(strings, personExp, ageExp) {
var str0 = strings[0]; // "that "
var str1 = strings[1]; // " is a "
// There is technically a string after
// the final expression (in our example),
// but it is empty (""), so disregard.
// var str2 = strings[2];
var ageStr;
if (ageExp > 99){
ageStr = 'centenarian';
} else {
ageStr = 'youngster';
}
return str0 + personExp + str1 + ageStr;
}
var output = myTag`that ${ person } is a ${ age }`;
console.log(output);
// that Mike is a youngster
Example taken from MDN. So you see there is a var output that is assigned with myTag (there is the function call) followed by the arguments passed to the function in a 'templatish' way. The words 'that' and 'is a' will be assigned to strings param of the function and the 2 expressions person and age will be assigned in declaration order to the next 2 params. It's simple.
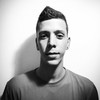
Jesus Mendoza
23,288 PointsString literals are easy
var sum = 1+1;
var sum2 = 2+2
var stringES5 = '1 + 1 is ' + sum + '.\n2 + 2 is ' + sum2 + '.';
console.log(stringES5);
// If you have a larger string you need to use multiple + to concatenate the whole string.
let stringES6 = `1 + 1 is ${sum}.
2 + 2 is ${sum2}.`;
console.log(stringES6);
Tagging is really difficult and i don't fully understand it.
Anthony Albertorio
22,624 PointsAnthony Albertorio
22,624 PointsTagged template literals are super useful when explained properly, especially for templating and dynamically changing a the DOM. I found a great explanation of tagged template literals here: https://www.youtube.com/watch?v=c9j0avG5L4c Kudos to Kyle Robinson Young for the video
Another explanation of template literals and their usage can be found here: https://www.youtube.com/watch?v=LTbnmiXWs2k&list=PL57atfCFqj2h5fpdZD-doGEIs0NZxeJTX Kudos to Ryan Christiani for the video