Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial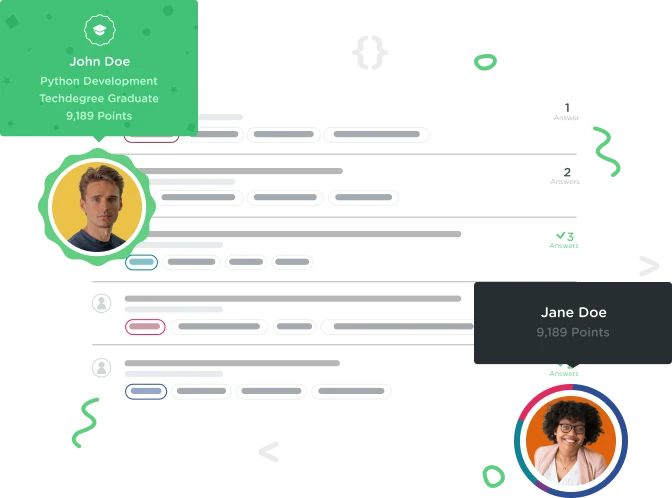
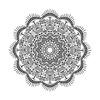
Arikaturika Tumojenko
8,897 PointsCan someone explain the code step by step?
I don't understand why the echo function attached to the second "if" nested statement executes since the "if" statement it's not true ($username != "Treehouse" evaluates to false, or I'm being wrong here?).
3 Answers

andren
28,558 PointsYou are correct that the nested if
statement will not run. The correct answer to the question is that nothing will be displayed.
Here is a breakdown of the code
<?php
$username = "Treehouse"; # Define username
if ($username) { # Check if $username is truthy (True)
if ($username != "Treehouse") { # Check if $username is not "Treehouse" (False)
echo "Hello $username"; # This does not run because the condition above is `False`.
}
} else { # Run if connected `if` statement above did not run
echo "You must be logged in"; # This does not run because the `if` statement above it ran.
}
The else
statement is attached to the first if
statement, not to the nested if
statement so it does not run since that first if
statement ran.
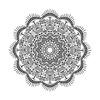
Arikaturika Tumojenko
8,897 PointsThank you, excellent answer, made things pretty clear to me (I think the video is a bit too vague for us to understand exactly how nested conditionals work).
I didn't take into consideration the third outcome - where the first "if" statement could be false because in my head it would always evaluate to true. Which takes me to another question: can you give me an example where the first if statement would evaluate to false?
$username = "Treehouse";
if($username == "Alana") == > would this code be considered a good example of a first if statement that would determine the program to jump to the ELSE statement?

andren
28,558 PointsYes a condition of $username == "Alana"
would lead the if
statement to not run, and cause the else
to run instead.
The thing that ultimately decides if an if
statement runs or not is whether it is passed the boolean
value true
or false
or something that ends up evaluating to true
or false
.
Comparisons like $username == "Alana"
are examples of code that evaluates to a Boolean
based on wheter or not the comparison ends up being correct or not. Since $username
is not equal to "Alana"
in your code snippet it would evaluate to false
which causes the if
statement to not run.
Here is a code example:
<?php
$username = "Treehouse";
if($username == "Alana") {
echo "Will not print";
} else {
echo "Will print";
}
You could also insert the Boolean
false
directly:
<?php
if(false) {
echo "Will not print";
} else {
echo "Will print";
}
Though that is rarely done with if
statements since it is pretty pointless.
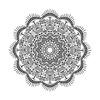
Arikaturika Tumojenko
8,897 PointsGreat. Thx again!
Arikaturika Tumojenko
8,897 PointsArikaturika Tumojenko
8,897 PointsSo... for echo to display "Hello $username", both "if" statements (let's call them the PARENT and the nested if statement) need to be true?
From what I understand we could have only these outcomes:
If this is the case, in which situation would the ELSE statement run - why did we write it in the first place if from what I understand the program will not get to it anyway?
andren
28,558 Pointsandren
28,558 PointsYes. If the first
if
statement isfalse
then nothing within its body (which includes the nestedif
statement) will run. And the same is true for the nestedif
statement, the contents of its body (theecho
statement) will not run unless it istrue
.There are three possible outcomes from the code:
if
statements aretrue
. This leads to"Hello $username"
being printed.if
statement istrue
. This leads to the situation described in my original answer.if
statement isfalse
. This leads to theelse
statement running. Since theelse
statement only runs when theif
statement it is attached to does not run.The reason why it was written was that it would be triggered if the first
if
statement wasfalse
, which would be the case if$username
contained theBoolean
false
or aFalsy
value. Like an empty string or something along those lines.That is not the case in the question code, but the purpose of the question is to see if you can figure out how PHP will navigate the
if
/else
statements, since that is a good test of your knowledge of howif
/else
statements work.