Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial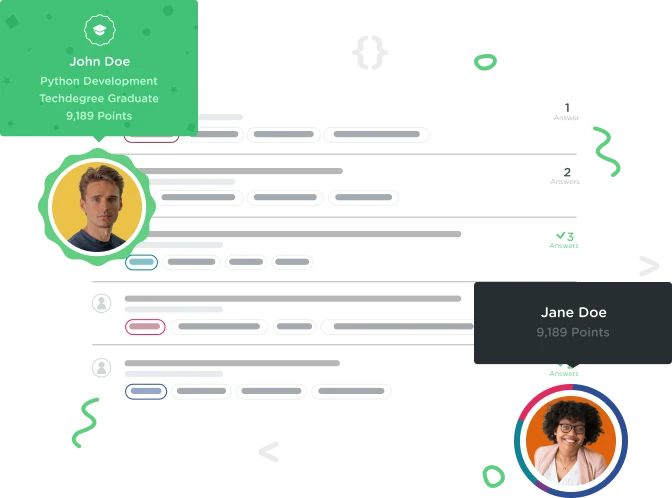

vamosrope14
14,932 PointsCan someone explain the code syntax and what the code means here?
.
3 Answers
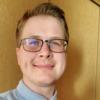
Alex Labins
20,826 PointsFor the first example:
const { foo } = bar;
This is just shorthand for
const foo = bar.foo;
It's extremely handy for multiple assignments all at once, too.
const yourModule = require('your-module');
const { app, router, parser, message } = yourModule;
The above code is is exactly the same as typing all this
const yourModule = require('your-module');
const app = yourModule.app;
const router = yourModule.router;
const parser = yourModule.parser;
const message = yourModule.message;
Here is the second example, where the assignment is basically just the other way around. I deliberately assigned them in a random order to demonstrate that it doesn't matter.
const bar = 0;
const so = 1;
const re = 2;
const mi = 3;
const foo = { so, bar, mi, re };
console.log(foo.bar); // 0
console.log(foo.mi); // 3
It's the exact same as typing this; just much more concise and less repetitive
foo.bar = bar;
foo.so = so;
foo.re = re;
foo.mi = mi;
As a fun fact, I initially had "bar do re mi" but do
is actually a reserved keyword!

Samuel Piedra
Full Stack JavaScript Techdegree Student 9,898 PointsThe syntax Chalkers is using in the video for assigning variables using the curly braces ( { variable } ) wrapped around the variable is called "Destructuring Assignment." As mentioned in the other post it is just a shorthand way of assigning an object's property value to a variable (this could be an object literal or array). When you use this syntax you are destructuring the object and grabbing value(s) from the object's properties.
For example in our "cards.js" file in our routes folder we import the json data from our "flashcardData.json" using:
const { data } = require("../data/flashcardData.json");
We can do this because the returned json gets treated like a regular JavaScript object with a single property named data. The data property is an object itself with two properties, the first being title and the second being cards. So instead of writing something like this:
// assign "flashcardJSON" to the returned json object. "flashcardJSON" now holds our json data.
const flashcardJSON = require("../data/flashcardData.json");
// now assign our data variable to the value of the data property in our "flashcardJSON" object
const data = jsonData.data
Because the json data from our "flashcardData.json" file has a property named data we can use the ES6 destructuring assignment syntax to assign the value of the json data's data property to a variable we want to name data, like so:
const { data } = require("../data/flashcardData.json");
We could just as easily call our variable something else, but we would need to make sure we identify which property's value we want to "destructure" and assign:
// assign the value of the "data" property from our json object to the variable "myFlashCardData"
const { data : myFlashCardData } = require("../data/flashcardData.json");
// now if I want to access the "title" and "cards" properties I can use:
console.log(myFlashCardData.title); // log the title
console.log(myFlashCardData.cards); // log the cards array
I really hope this helps. "Destructuring Assignment" is an simpler way to assign property values from object's to another variable or variables. I found this MDN link very helpful!

Christine Treacy
Full Stack JavaScript Techdegree Graduate 18,412 PointsAlex Labins & Samuel Piedra Thank you for your thorough explanations! These definitely helped me understand this concept better.
Seokhyun Wie
Full Stack JavaScript Techdegree Graduate 21,606 PointsSeokhyun Wie
Full Stack JavaScript Techdegree Graduate 21,606 PointsAlex Labins It's such a helpful answer, but no appreciation here, so I leave one up-vote here. Thanks Alex.