Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial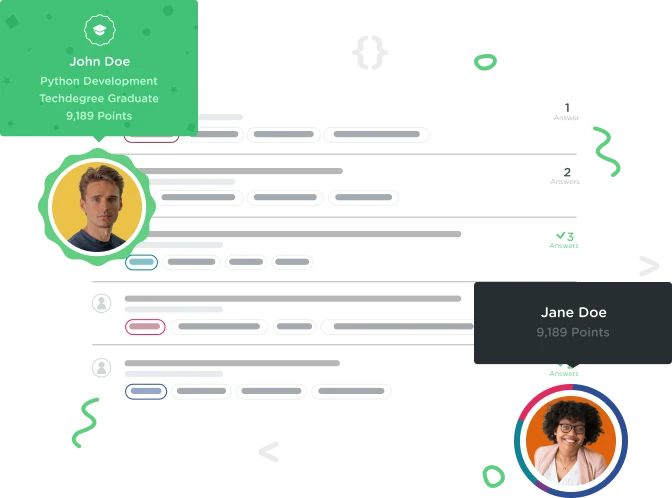
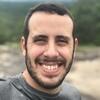
Peter Correa
17,155 PointsCan someone explain the following code?
I understand that the code is intializing the Contacts struct. However I need clarification on what's going on inside the init? method, more specificaly the use of the subscripts with the parameter "dictionary."
struct Contact {
let firstName: String
let lastName: String
let phone: String
let email: String
let street: String
let city: String
let state: String
let zip: String
let image: UIImage?
var isFavorite: Bool
}
extension Contact {
struct Key {
static let firstName = "firstName"
static let lastName = "lastName"
static let phone = "phoneNumber"
static let email = "email"
static let street = "streetAddress"
static let city = "city"
static let state = "state"
static let zip = "zip"
static let image = "avatarName"
}
//this is where I am stuck...
init?(dictionary: [String: String]) {
guard let firstNameString = dictionary[Key.firstName],
let lastNameString = dictionary[Key.lastName],
let phoneString = dictionary[Key.phone],
let emailString = dictionary[Key.email],
let streetString = dictionary[Key.street],
let cityString = dictionary[Key.city],
let stateString = dictionary[Key.state],
let zipString = dictionary[Key.zip] else { return nil }
self.firstName = firstNameString
self.lastName = lastNameString
self.phone = phoneString
self.email = emailString
self.street = streetString
self.city = cityString
self.state = stateString
self.zip = zipString
if let imageName = dictionary[Key.image] {
self.image = UIImage(named: imageName)
} else {
image = nil
}
isFavorite = false
}
}
1 Answer
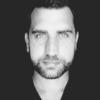
Jhoan Arango
14,575 PointsHello,
It's simple really, the init is a fail-able initializer, that takes a dictionary as its parameter. So inside of it, you have a guard that if any of the information is missing, it will fail to initialize the struct.
// We have a dictionary
let someCarDictionary: [String : String] = ["fiesta" : "Ford Fiesta" , "mustang" : "Ford Mustang"]
// This is how we retrieve a value from a dictionary, by passing it the key. ( Key Value )
let car = someCarDictionary["fiesta"]
print(car) // Will print "Ford Fiesta"
So in the init, all it's doing is passing keys for the respective value to be able to initialize all its properties.
Hope that helps you a bit.
If you need a more detail answer let me know.
Good luck
Peter Correa
17,155 PointsPeter Correa
17,155 PointsThanks Jhoan! Really helped!