Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial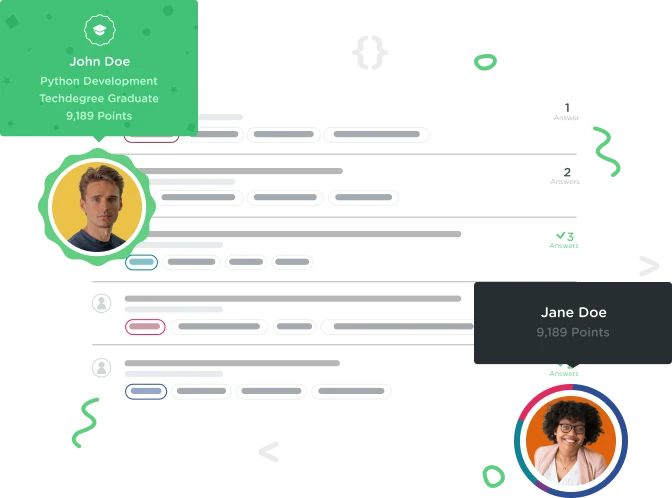

Michael Zaro
13,196 PointsCan someone explain the syntax of numberOfRowsInSection and cellForRowAtIndexPath?
I know that this has something to do with some bleed-over from Objective-C, but Pasan kind of glances over the fact that this syntax is very different from what we've seen so far in Swift programming. It appears like the MasterViewController is overriding the tableView()
method over and over, but in talking with a colleague of mine, the better way to think of it is that tableView is an instance of the UITableView class, and what we're really doing is overriding the numberOfRowsInSection
and cellForRowAtIndexPath
methods on the tableView instance.
The confusing part is where it shows override func tableView(...)
because it looks like it's overriding some tableView method.
Anyway, long story short - I'd like an explanation of the syntax of:
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return fruits.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Cell", forIndexPath: indexPath) as! UITableViewCell
let fruit = fruits[indexPath.row]
cell.textLabel!.text = fruit
return cell
}
override func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the specified item to be editable.
return true
}
Particularly how it is that the method is actually what looks like the second parameter of some tableView
function, and what the space is doing between that apparent parameter and the next word following it, such as in cellForRowAtIndexPath indexPath: NSIndexPath
1 Answer
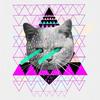
Stepan Ulyanin
11,318 PointsHi, The delegate of a UITableView object must adopt the UITableViewDelegate protocol. Optional methods of the protocol allow the delegate to manage selections, configure section headings and footers, help to delete and reorder cells, and perform other actions.
So the methods you override are delegate methods to manage the cells and everything that is connected with your table view.
As for the argument passing:
Function parameters have both an external parameter name and a local parameter name. An external parameter name is used to label arguments passed to a function call. A local parameter name is used in the implementation of the function.
func someFunction(externalParameterName localParameterName: Int) {
// function body goes here, and can use localParameterName
// to refer to the argument value for that parameter
}
This external parameter names are there for your convenience, same principle is used in objective-c:
- (void)functionNameAndParameterOneName:(id)parameterOne andParameterTwoName:(id)parameterTwo {
//some stuff
}