Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial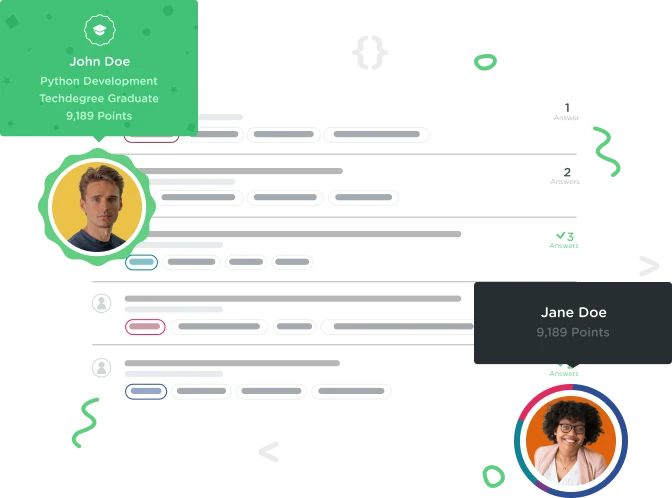

Jamshaid Ali
189 PointsCan someone explain this a little more i didnt quite understand it
Can someone please explain the video a little more and how the colons and equal equal signs work.
Can you also elaborate more on the if and else and elif a bit more to please.
3 Answers
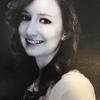
Megan Amendola
Treehouse TeacherHi! Conditionals are pieces of code that run if something is True
. You can think of them as a kind of decision tree. Let's stick with the weather for an example.
If it's raining outside, then I need to grab an umbrella. If It's snowing, then I'll need a warm coat. Otherwise, I can bring a light jacket with me just in case.
Let's translate this into code!
if condition:
# do something if this is true
# this code must be after the colon :
# and indented inside to run
elif condition:
# do something if this condition is true
# this code must be after the colon :
# and indented inside to run
else:
# if neither of the above two conditions is true, then run this
# this code must be after the colon :
# and indented inside to run
This is what conditionals look like in Python. You use ==
equals, >
greater than, <
less than, >=greater than or equal to,
<=` less than or equal to create conditionals. There are other ways to create conditionals but these are the basics
The code you want to run when this condition is true is placed 'inside' the statement by closing the statement with a colon and then indenting the code on the following lines. Anything indented after the statement is considered a part of the conditional.
if weather == 'raining':
# grab an umbrella
# maybe grab some rain boots
# even though there is an empty line, this is still indented and therefore a part of the above conditional
elif weather == 'snowing':
# grab a warm coat
else:
# grab a light jacket
Let's practice this with some numbers:
num = 5
if num < 3:
print("It's less than 3")
elif num < 6:
print("It's less than 6")
else:
print("It's neither less than 3 nor less than 6")
In the above code, what will be printed out?
Let me know if you still need some more explanation

Jamshaid Ali
189 PointsAhh ok great also i have 2 more questions, in the video Craig uses ".format" and he use "{}" as place holders can you explain what these two are and what they do
P.S thank you megan you have helped me alot!
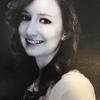
Megan Amendola
Treehouse TeacherStrings can be formatted. This is where you are able to pass in information to be placed inside of a string.
animal = 'dog'
# here you are formatting the string to place the string dog at the end of the sentence
print('My favorite animal is a {}'.format(animal))
>>> My favorite animal is a dog
# there are a few other ways to write this out
print('My favorite animal is a {pet}'.format(pet = animal))
print('My favorite animal is a {0}'.format(animal))
# You can place almost anything inside of a string using string formatting like a number
price = 5.99
# this will convert the float above into a string and place it inside of this string
print('The price is ${}'.format(price))
>>> The price is $5.99
# there is also a newer way of formating strings called f-strings
price = 5.99
# this does the same thing you just start with an f before the string and place the variable inside the curly brackets
print(f'The price is ${price}')
>>> The price is $5.99
# it also doesn't have to be a variable
print(f'The price is ${5.99}')

Jamshaid Ali
189 Pointsahh ok thank you
Jamshaid Ali
189 PointsJamshaid Ali
189 Pointswhat is a indented ?
Megan Amendola
Treehouse TeacherMegan Amendola
Treehouse TeacherJamshaid Ali, indented is when you have either a tab or 4 spaces at the beginning of a line. Everything in Python works with indentations. In the example above, the
print()
statements are indented by 4 spaces and are therefore inside of theif
,elif
, andelse
statements.