Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial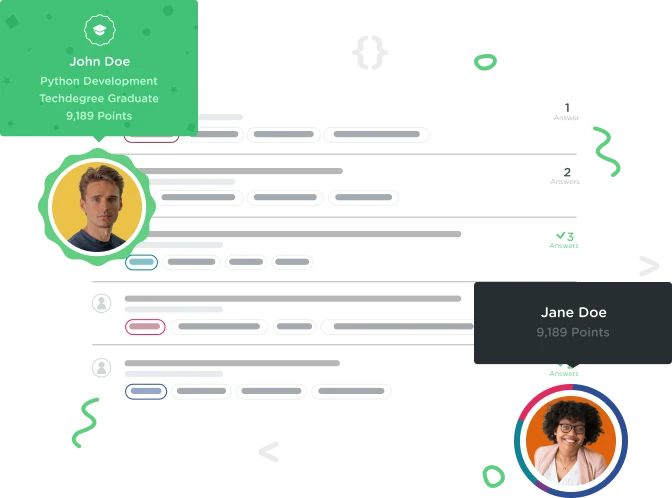

Dylan Carter
4,780 Pointscan someone explain this in more detail?
Okay so I get all the individual concepts for the most part but I get confused on when its all put together like this. can someone please go through this code step by step and explain in full like your talking to someone NEW to doing this, treat me like I'm in kindergarden with your responses please. I cannot follow craig just explaining each individual step like extremely briefly.
like the for loop for the treets, we look for every Treet object that we are giving the variable name treet, inside of the array of Treet objects we previously made called treets. so there are many different Treet objects inside of the source of the left of the for loop, treets (which is our array of treets again), and what this is doing is looping though each object in that array, using the variable name treet to refer to each one that gets looped though. then we will open our code block where we will put in the steps of what needs to be done to each one of these treets....
if someone could explain these steps like that to me I would GREATLY appreciate it. I don't expect you to write me a book like that but along those lines would be very helpful. I just cant follow when craig will take my explaination above and say "ok were looking for treets in treets."
Map<String, List<Treet>> treetsByAuthor = new HashMap<String, List<Treet>>();
for (Treet treet : treets) {
List<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
if (authoredTreets == null) {
authoredTreets = new ArrayList<Treet>();
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
}
authoredTreets.add(treet);
}
System.out.printf("Treets by author: %s %n", treetsByAuthor);
System.out.printf("Treets by nickrp: %s %n", treetsByAuthor.get("nickrp"));
}
}
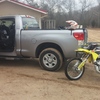
Ryan Ruscett
23,309 PointsFormatted the code for you.

Dylan Carter
4,780 PointsOk thanks I guess
1 Answer
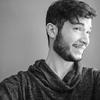
Damian Adams
21,351 PointsThis answer is coming in a little late but hopefully it'll help someone else that's stuck in the same place as well (:
Alright, let's go over this through a series of six easy steps:
First:
Map<String, List<Treet>> treetsByAuthor = new HashMap<String, List<Treet>>();
We are creating a Map data structure with types String and List (a List of type Treet).
Second:
for (Treet treet : treets)
We are iterating through the array of objects of type Treet we already have, which we stored in the variable treets.
Third:
List<Treet> authoredTreets
We create the List that we intend to store as our Map value.
Fourth:
List<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
We use the Map method get(), which goes into the Map we created and checks to see if the author of the current Treet we are iterating through has already been stored in our Map.
Following this, we have two possible outcomes:
Outcome 1:
if (authoredTreets == null) {
authoredTreets = new ArrayList<Treet>();
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
}
If it is a new author, it returns a value of "null" which is fine because we now know that this author is a new Key that we want to add to our Map. Now we move on into our conditional if statement (which is true in this outcome), we create a new ArrayList of type Treet, and we put this empty list as our value for our current author in our Map. We will add the treet into this List in step six.
Outcome 2:
If it is an author that we previously added into our Map, the method get() returns the List stored in this value. Which is fine because now that this List has been handed over to us, we can go on ahead and add a new author Treet to this List.
Fifth:
authoredTreets.add(treet);
Whichever one of the outcomes resulted to be the case, we go on ahead and add the Treet into our List. This List has already been stored into our Map, so it is "updated" automatically.
It does this through the use of object references (which you might like to read up on if you'd like to understand this further).
Sixth:
System.out.printf("Treets by author: %s %n", treetsByAuthor);
System.out.printf("Treets by nickrp: %s %n", treetsByAuthor.get("nickrp"));
Now we print out this Map to the screen. And afterwards we go on ahead and print a List of Treets written by the author (our key) "nickrp".
I hope this helped clear everything up!
Dylan Carter
4,780 PointsDylan Carter
4,780 Pointsoh and one more comment, why are we returning a list of treets for the value? shouldn't it be the author as a string and then the full treet as just a treet object? so the map would look like @john=johns full treet entry?