Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial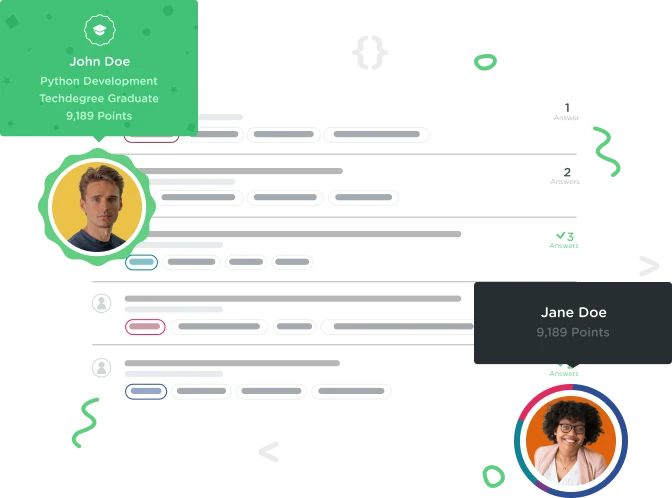

Alex Flores
7,864 PointsCan someone explain this list function to me?
I'm reading through Eloquent JavaScript and I'm doing the examples, but this one totally stumped me. I have no idea how/why this works the way it does. The goal of the function it to take a list as an argument and return the list index specified by the other argument.
<script>
function nth(list, n) {
if (!list)
return undefined;
else if (n == 0)
return list.value;
else
return nth(list.rest, n - 1);
}
</script>
So this is a recursive function and it's going through each list index, one by one and it's stopping once n = 0. My question is, why is the compiler reading it like this (one by one down the list). Isn't there an easier way to call a list value?
1 Answer
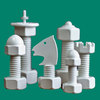
Steven Parker
230,274 PointsThis function appears to be designed to work with a particular kind of list known as a linked list. There's no index ([]) operation for this kind of list, so the only way to access a particular element is to search through the chain of elements as this function does.
This would have been a bit clearer if you had included the definition of the list the function works with, but it would appear that each element contains a value named value and a reference to the next element named rest.
The complexity is due to the type of list. Other types can be accessed in simpler ways. Hopefully, there's an explanation of why and when you might use this type of list in the place where you found this code.