Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial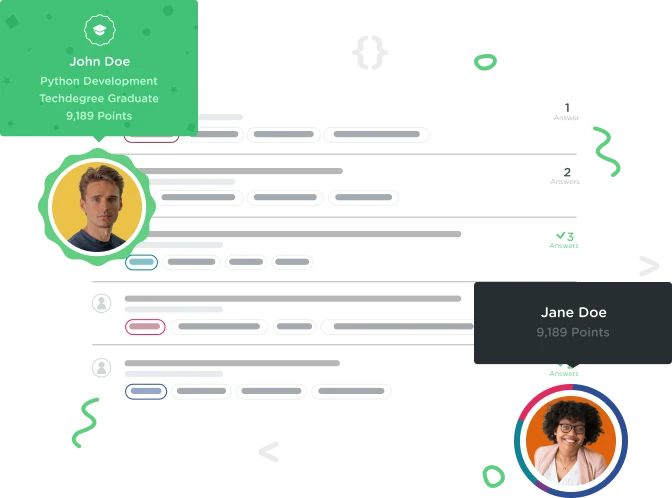

Jonathan Franco
Courses Plus Student 1,021 PointsCan someone explain to me in detail about how this script is running?
travel_expenses = [ [5.00, 2.75, 22.00, 0.00, 0.00], [24.75, 5.50, 15.00, 22.00, 8.00], [2.75, 5.50, 0.00, 29.00, 5.00], ] print("Travel Expenses") week_number = 1 for week in travel_expenses: print("* Week #{}: ${}".format(week_number, sum(week))) week_number += 1
i have a basic understanding about what is going on, but i feel like i need a bit more clarity with multi dimensional lists. i understand how rows and columns work in this list, but how about the lines of code under it?
I appreciate a response in advance!!
1 Answer
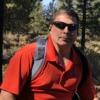
Mark Kastelic
8,147 Points# travel_expenses is a list of lists. each sublist presumably contains the expenses for each
# day of a week as there are five values.
travel_expenses = [ [5.00, 2.75, 22.00, 0.00, 0.00], [24.75, 5.50, 15.00, 22.00, 8.00], [2.75, 5.50, 0.00, 29.00, 5.00], ]
print("Travel Expenses")
# initialize the week_number variable to track the iteration through the list of weeks (sublists).
week_number = 1
# the for loop will iterate through each item (i.e., sublist) in the list of weeks (travel_expenses)
for week in travel_expenses:
# for each "week" or sublist in travel_expenses, print a label indicating the "Week #" followed
# by the sum or total of the five days of expenses for that week. Note: the curly brackets "{}"
# are placeholders for the values contained in the variables/expressions: "week_number" and
# "sum(week)." "week_number" starts at 1 and then before the next week sublist is iterated,
# "week_number" gets incremented by 1 with the statement "week_number += 1. "sum(week)" evaluates
# to the sum or total of the five daily expense values in each week (Note: the for loop uses the
# variable "week" to iterate through travel_expenses, so each time through the loop, "week" is
# equal to one of the sublists contained in travel expenses.
print("* Week #{}: ${}".format(week_number, sum(week)))
week_number += 1
I apologize if this explanation is too verbose! As a final observation, I prefer to use "enumerate" to iterate through lists when I need to access the index of the item (in this case to extract the number of the week). The code below shows this implementation which simplifies things a little:
travel_expenses = [ [5.00, 2.75, 22.00, 0.00, 0.00], [24.75, 5.50, 15.00, 22.00, 8.00], [2.75, 5.50, 0.00, 29.00, 5.00], ]
print("Travel Expenses")
for index, week in enumerate(travel_expenses):
print("* Week #{}: ${}".format(index + 1, sum(week)))
Note: since indexing in Python starts at 0, (i.e., the first week of data can be accessed with: travel_expenses[0]), we need to offset the index variable by 1