Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial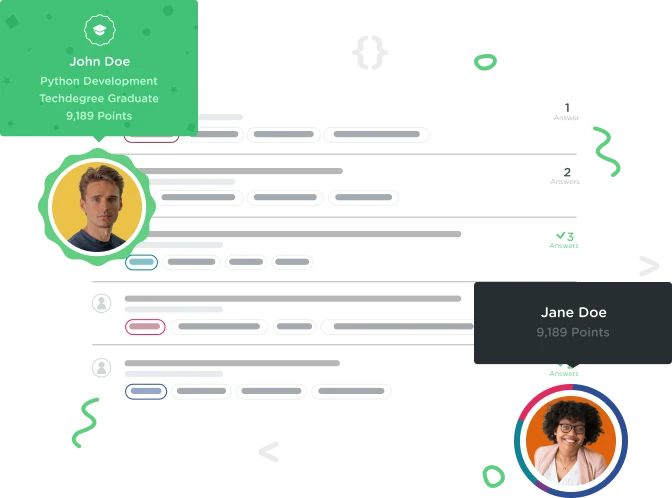

Mathew Yangang
4,433 PointsCan someone explain to me the main difference between using def__mul__ and def__rmul__ when adding to class
Can someone explain to me the main difference between using def_mul_ and def_rmul_ when adding to class?
1 Answer
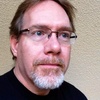
Chris Freeman
Treehouse Moderator 68,457 PointsGood question! The __mul__
method is needed if your class will be used in a numeric context on the left side of the multiplication operator. The __rmul__
method is needed if your class will be used in a numeric context on the reflected side, that is the right side of the multiplication operator.
Post back if you need more help. Good luck!!
Ernestas Petruoka
1,856 PointsErnestas Petruoka
1,856 PointsCan you give some examples? I completely lost in object oriented python course and that super anoying so please help me :(
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsHi Ernestas,
The methods
__mul__
and__rmul__
work in the same fashion as__add__
and__radd__
. Without__mul__
, an object could not be used on the left-side of a multiplication operator. Without__rmul__
, an object could not be used on the right-side (or reflected side) of a multiplication operator.Say there is a class called
Dice
that can be used in a numeric context, that is, with operators addition (+) and multiplication (*). I've created a version of the D6 and Die classes where each method will print as it runs. You may follow the flow of methods for addition and multiplication below.For coding simplification, in addition, the
__radd__
method calls the__add__
method and, in multiplication, the__radd__
method calls the__add__
method.Post back if you have more questions. Good luck!!