Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial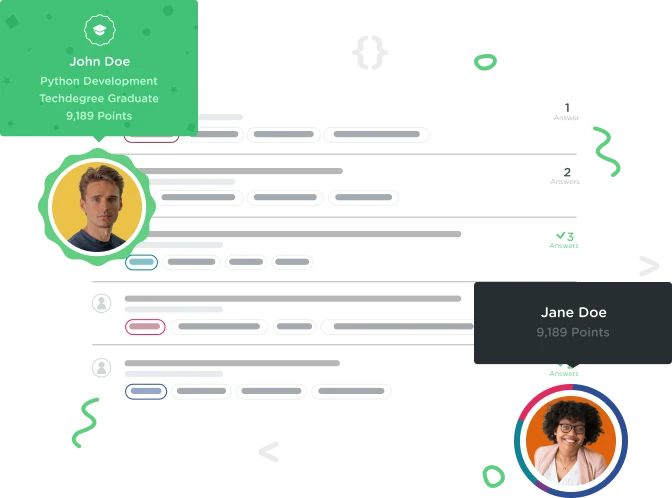

Michael Hampshire
12,206 PointsCan someone explain to me why my program wouldn't catch the exception?
I'm on the final code challenge for C# and I followed along no problem with the class. When I got to the end though I was having problems with the code compiling. I don't know if it was just the work spaces or what but I write all my code in Visual Studio and then just copy and paste it in.
But my main problem is that I can't get the program to catch my exception. It catches it after it crashes, but won't in the program. I can type in how every many "Yays" I want and it prints them and ends the program, but won't throw an exception when I input something besides an Int. Here is my code:
class Program {
static void Main(string[] args)
{
var looped = 0;
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
var times = int.Parse(entry);
while (looped < times)
{
try
{
Console.WriteLine("Yay!");
looped += 1;
continue;
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
Console.ReadLine();
}
}
}
P.S: I left out the namespace part at the top, as well as the using system.
1 Answer
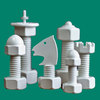
Steven Parker
231,271 PointsYour structure is not quite correct. The purpose of the try and catch is to validate that the input is a number, so for it to do that the try block must enclose the line where you call int.Parse (and not be inside the loop). Some other things to keep in mind are:
- Your program must ask for and accept input ONE TIME ONLY (so only one Readline)
- If the input validates, your program will perform exactly as in Task 1
- If the input does not validate, it will print the error message and exit
- since the parsing will be inside the try, the times variable must be declared separately from the assignment