Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial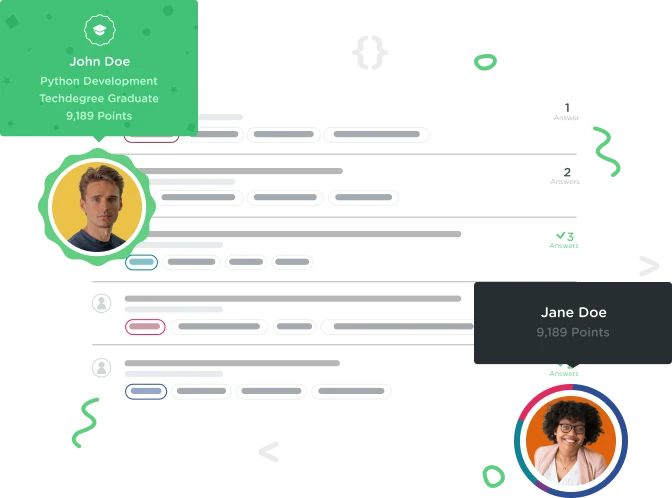

Parimal Raghavan
Courses Plus Student 1,940 PointsCan someone explain what this for loop does?
for key, values in kwarg.items():
setattr(self, key, value).
What exactly does this do?
[MOD: added ```python markdown formatting]
1 Answer
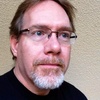
Chris Freeman
Treehouse Moderator 68,423 PointsWhen a function is defined using **kwargs
as a parameter, it will accept an arbitrary number of keyword arguments. These arguments are assigned in a dict-like object where the argument names are the dict keys and the argument values are the dict values. The code parses the keyword arguments and set local object attributes.
# for each key and value pair in the kwargs dict
for key, values in kwargs.items():
# set the local attribute of this object
# where the attribute name is the 'key' and the attribute value is the 'value'
setattr(self, key, value).
Post back if your need more details
Nursultan Bolatbayev
16,774 PointsNursultan Bolatbayev
16,774 PointsBut why we cant do it without .items() function? For example when we run a loop for dictionary we dont use it, right?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsUsing
.items()
is one of many ways to iterate over a dict. When not explicitly using a method, as in:for item in some_dict:
This default method is to iterate over the keys of the dict. The equivalent statement written explicitly:
for item in some_dict.keys():
The third method available is
.values()
which iterates over the values in a dict.Back to the question, using
.items()
is a matter of convenience to retrieve both the key and value together. The solution could be rewritten as:Nursultan Bolatbayev
16,774 PointsNursultan Bolatbayev
16,774 PointsChris Freeman , thanks. Now it is clear.