Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial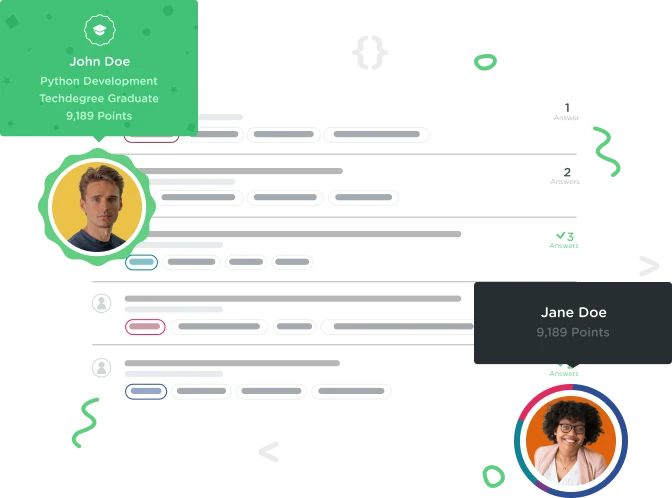

Jennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsCan someone explain why my jar isn't filling with a random number of items?
I'm struggling with getting my fillJar() method to work correctly. Can someone explain to me what I'm doing wrong and how to fix it without providing me the actual code? I need to learn that myself but I'm still having troubling conceptualizing the steps to take to make my method work.
Main Class
public class GuessingGame {
public static void main(String[] args) {
System.out.println("ADMINISTRATOR SETUP\n********************");
System.out.println("GUESSING GAME: Guess how many items are in the jar.\n\nSelect the type of items, and how many can reasonably fit in a jar.\nSound good? Okay let's begin!\n");
if(args.length != 2) {
Jar jar = new Jar();
jar.fillJar();
}
}
}
Jar Class
import java.util.Scanner;
import java.util.Random;
public class Jar {
private String itemName;
private String maxItems;
public Jar() {
Scanner scanner = new Scanner(System.in);
System.out.println("What type of item?");
this.itemName = scanner.nextLine();
System.out.println("What is the maximum amount of " + itemName + " ?");
this.maxItems = scanner.nextLine();
System.out.print("A new jar has been created and filled with " + itemName + ".\n");
System.out.println("PLAYER\n********************\nHow many " + itemName + " are in the jar?\nPick a number between 1 and " + maxItems);
}
public void fillJar(randomNumber) {
Random randomNumber = new Random();
randomNumber = randomNumber.nextInt(Integer.parseInt(maxItems));
}
}
1 Answer
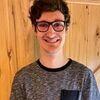
Trent Christofferson
18,846 PointsI would suggest storing the number of items in the jar in a variable and when you call fillJar() you change the value of that variable. Then create a method that checks to see if their guess is equal to the number of items in the jar. Currently, your fillJar() method does nothing. It just takes parameter randomNumber generates a random number and does nothing with it, by the way, I think it is supposed to be int randomNumber as a parameter. That is where you would store it to the variable I said you should create.