Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial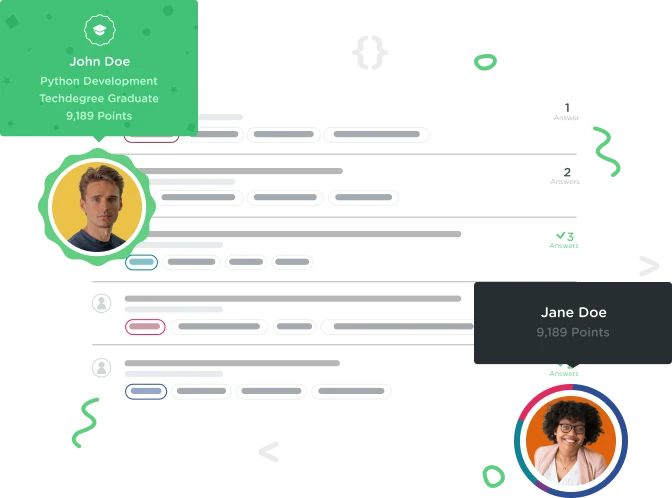

mfod
1,708 PointsCan someone explain why the parameter inside the constructor needs to be passed inside the member variable?
For some reason I ALWAYS have a difficult time understanding constructors and how to use them.
public PezDispenser(String characterName) { mCharacterName = characterName; /* what does this mean exactly*/ }
Please help.
2 Answers
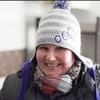
Dina Climatiano
10,862 PointsThe reason we pass parameters through the constructor into the member variables is because we don't want to expose private member variables outside. Meaning: we don't want our class to be used like this: PezDispenser pd = new PezDispenser(); (First initializing the instance without setting any values in the member variables) pd.mCharacterName = "whatever"; (Accessing member variables directly and freely setting values to them)
There are several reasons why this is wrong practice (in this case): a. We set the mCharacterName to private - so we physically will not be able to use it like that. b. If we were setting it to public - who's to stop other people from changing that member variable in other random places in the code (meaning: misusing our class).
Assuming we don't want the character name to be changed after initialization we can hard code the name we want in the class, thus naming every Dispenser from here on out the same name that's hard coded in the class. Like this:
public class PezDispenser { private String mCharacterName = "Yoda";
//The rest of the code }
But... suppose we want every instance of the Pez Dispenser to be different. A constructor is merely the function that initializes an instance of the Dispenser (which is different than the class - which is what a Dispenser should include and how it behaves). The parameter passed inside the constructor is our chosen character name. But passing the parameter into the constructor alone is not enough - you need to "tell" the parameter where it goes. In this case - we want our chosen character name to go inside the appropriate place in the Pez Dispenser "mold" (the class we created) - which is the mCharacterName member variable. So within the constructor we appropriate the use of every parameter passed to its' appropriate place in the class.
Also - the name of the parameter passed does not matter. It's called "characterName" for convenience reasons here (so that it's obvious that it goes into mCharacterName), but it could have been called "firstParam" or "name" or whatever you want. It doesn't matter, as long as you send the received data (parameters passed into the constructor) into its' correct place in the class. The constructor could have looked like this:
public PezDispenser(String whatever) { mCharacterName = whatever; }
and worked just as well, it's just a lot less readable...
Understanding the difference between classes and instances is tricky at first, but you'll catch on soon enough. I hope I've been clear.

raajiabraham
2,609 PointsConstructors that have no parameters always have the (class ) member variables with default values during run time. That means, a PezDispenser object will always have a default value for mCharacterName variable.
Constructors with parameters can set a different value for the member variables at run time. That is, all the PezDispenser objects can have different values for mCharacterName at run time. For this reason the parameter inside the constructor needs to be passed inside the member variable.
mfod
1,708 Pointsmfod
1,708 PointsWow! Thanks a lot! It took me a whole year to finally understand that... you taught me in three paragraphs! :)