Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial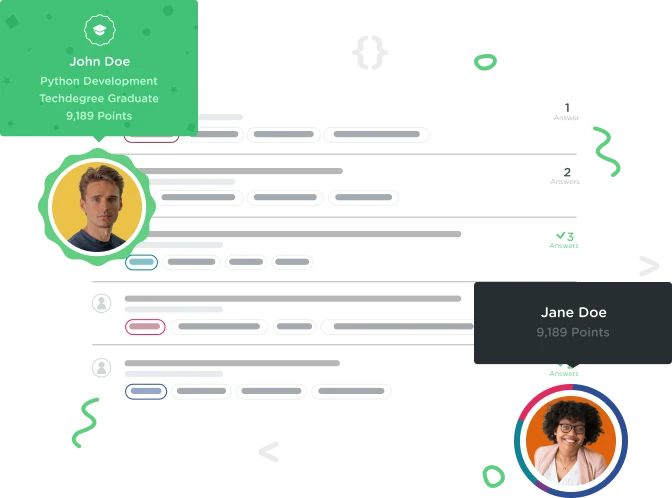

Ermin Bicakcic
5,144 PointsCan someone explain why this for loop does not take into account newly added list items?
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const listUl = listDiv.querySelector('ul');
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
const removeItemButton = document.querySelector('button.removeItemButton');
const lis = listUl.children;
const firstListItem = listUl.firstElementChild;
const lastListItem = listUl.lastElementChild;
addElement = (type, className, textContent) => {
const element = document.createElement(type)
element.className = className;
element.textContent = textContent;
return element;
}
function attachListItemButtons (li) {
let up = addElement('button', 'up', 'Up');
li.appendChild(up);
let down = addElement('button', 'down', 'Down');
li.appendChild(down);
let remove = addElement('button', 'remove', 'X');
li.appendChild(remove);
}
testForFirstLastItem = () => {
for (let i = 0; i < lis.length; i += 1) {
if (lis[i] == firstListItem) {
console.log(lis[i]);
lis[i].getElementsByClassName('up')[0].style.visibility = 'hidden';
} if (lis[i] == lastListItem) {
console.log(lis[i]);
lis[i].getElementsByClassName('down')[0].style.visibility = 'hidden';
} else if (lis[i] != firstListItem && lis[i] != lastListItem) {
console.log(lis[i]);
lis[i].getElementsByClassName('up')[0].style.visibility = 'visible';
lis[i].getElementsByClassName('down')[0].style.visibility = 'visible';
}
}
}
for (let i = 0; i < lis.length; i += 1) {
attachListItemButtons(lis[i]);
}
// Button interaction (Up, Down and Remove)
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
} if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
} if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(nextLi, li);
}
}
testForFirstLastItem();
}
});
// Hide Show
toggleList.addEventListener('click', () => {
if (listDiv.style.display == 'none') {
toggleList.textContent = 'Hide list';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show list';
listDiv.style.display = 'none';
}
});
// Change text on click
descriptionButton.addEventListener('click', () => {
descriptionP.innerHTML = descriptionInput.value + ':';
descriptionInput.value = '';
});
// Add new li through input
addItemButton.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
attachListItemButtons(li);
ul.appendChild(li);
addItemInput.value = '';
testForFirstLastItem();
});
testForFirstLastItem();
2 Answers
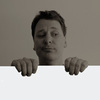
Sean T. Unwin
28,690 PointsYou're going to have to reassign a portion of the variables after you append()
items because you're only tracking the DOM at the point of declaration.
Change the const
to let
or var
on the initial declarations then reassign as needed in the attachListItemButtons()
function as well as addItemButton.addEventListener()
to always have the most recent DOM.
Another option for the reassignments would be to use a MutationObserver (link) in order to keep your code a little DRY-er. This is more complex, but could be more beneficial.

Ermin Bicakcic
5,144 PointsThank you for your answer. it is appreciated!
Ermin Bicakcic
5,144 PointsErmin Bicakcic
5,144 PointsI always get elements that were recorded in the first place. never updated structure.