Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial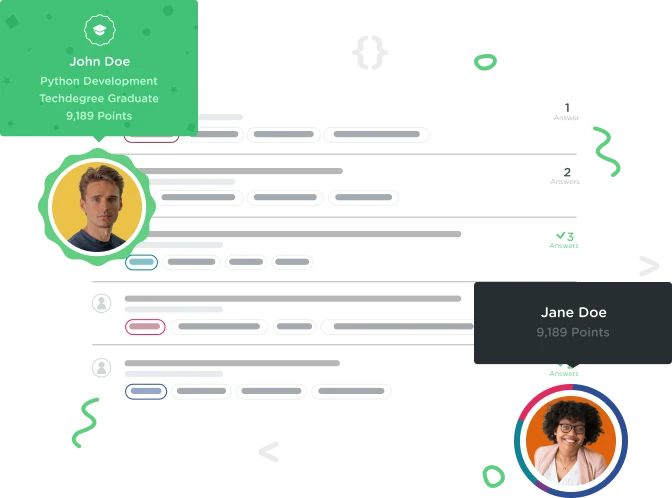

Nathan McGinley
4,627 PointsCan someone explain why this works?
So I know this code works but I don't understand how it does:
def loopy(items):
for item in items:
if item[0] == "a":
continue
print(item)
Shouldn't it be:
def loopy(items):
for item in items:
if items[0] == "a":
continue
print(item)
items is the list(array) and item is the variable holding the value for each index and as such isn't a list so how does this work, I'm so confused.
1 Answer
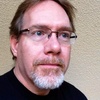
Chris Freeman
Treehouse Moderator 68,425 Pointsitems[0]
is the first element of the list passed in as an argument. It will have the same value for the entire function execution.
item[0]
is the first character of the loop variable item
. item
points to each successive element in the argument items
as the loop iterates over items
.
A string offset and slicing notation uses the same index notation as a list.
Mixing these concepts, you can use items[0][0]
to get the first character of the first word in the wordlist items
.
Nathan McGinley
4,627 PointsNathan McGinley
4,627 PointsAh thanks, makes sense now! I got all muddled up.
mykolash
12,955 Pointsmykolash
12,955 PointsHi Nathan McGinley.
Yep, Chris Freeman explained it. The only thing concerning: "Mixing these concepts, you can use items[0][0] to get the first character of the first word in the wordlist items."
Yes, if you need " the first character of the first word in the wordlist items" you can use [0][0]. But. If you don't know specific item #? Then you can use iterator (like "i" - like [i][j]) - like all "crappy" C++/Java/... languages do. And this trick is needed in Python too, but rather rarely. More often we can use other cool Pythonic tricks to solve iteration needs - like enumerate, for example.
But I think you've already studied it. During last 7 months. Before I've posted this comment. ^_^
Pls, excuse me if I'm just confusing you.