Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial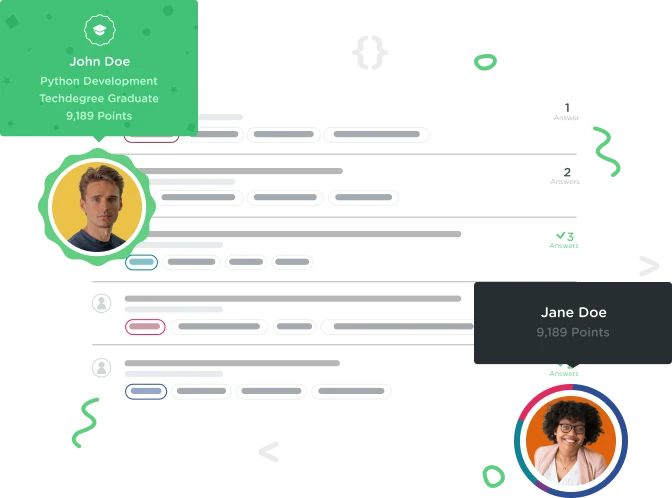

Elvis Chitsungo
1,817 PointsCan someone help.
Can someone help
public class Spaceship{
public String shipType;
public String getShipType() {
return shipType;
}
public void setShipType(String shipType) {
this.shipType = shipType;
}
6 Answers
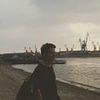
Calin Bogdan
14,921 PointsThere is a ‘}’ missing at the end of the file, the one that wraps the class up.
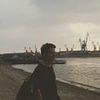
Calin Bogdan
14,921 PointsOne issue would be that shipType property is public instead of being private.

Elvis Chitsungo
1,817 PointsU mean changing this ''public void'' to private void.
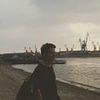
Calin Bogdan
14,921 PointsThis is correct:
public class Spaceship{
private String shipType;
public String getShipType() {
return shipType;
}
public void setShipType(String shipType) {
this.shipType = shipType;
}
The only change you have to make is public String shipType -> private String shipType.
Fields (shipType) have to be private, their accessors (getShipType, setShipType) have to be public.

Elvis Chitsungo
1,817 PointsAfter changing to private in now getting below errors.
./Spaceship.java:9: error: reached end of file while parsing } ^ JavaTester.java:138: error: shipType has private access in Spaceship ship.shipType = "TEST117"; ^ JavaTester.java:140: error: shipType has private access in Spaceship if (!tempReturn.equals(ship.shipType)) { ^ JavaTester.java:203: error: shipType has private access in Spaceship ship.shipType = "TEST117"; ^ JavaTester.java:205: error: shipType has private access in Spaceship if (!ship.shipType.equals("TEST249")) { ^ 5 errors
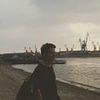
Calin Bogdan
14,921 PointsThat's because you have to use ship.getShipType() to get its value and ship.setShipType(type) to set its value. It is recommended to do so to ensure encapsulation and scalability.
Here are some solid reasons for using encapsulation in your code:
- Encapsulation of behaviour associated with getting or setting the property - this allows additional functionality (like validation) to be added more easily later.
- Hiding the internal representation of the property while exposing a property using an alternative representation.
- Insulating your public interface from change - allowing the public interface to remain constant while the implementation changes without affecting existing consumers.
- Controlling the lifetime and memory management (disposal) semantics of the property - particularly important in non-managed memory environments (like C++ or Objective-C).
- Providing a debugging interception point for when a property changes at runtime - debugging when and where a property changed to a particular value can be quite difficult without this in some languages.
- Improved interoperability with libraries that are designed to operate against property getter/setters - Mocking, Serialization, and WPF come to mind.
- Allowing inheritors to change the semantics of how the property behaves and is exposed by overriding the getter/setter methods.
- Allowing the getter/setter to be passed around as lambda expressions rather than values.
- Getters and setters can allow different access levels - for example, the get may be public, but the set could be protected.

Elvis Chitsungo
1,817 PointsThis is now becoming more complicated, i don't know much about java. But with the first one i was only having one error. i am referring to my first post. Let me re-type it again
./Spaceship.java:9: error: reached end of file while parsing } ^ 1 error

Elvis Chitsungo
1,817 PointsCalin Bogdan thanks, u have managed to give me the best answer.