Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial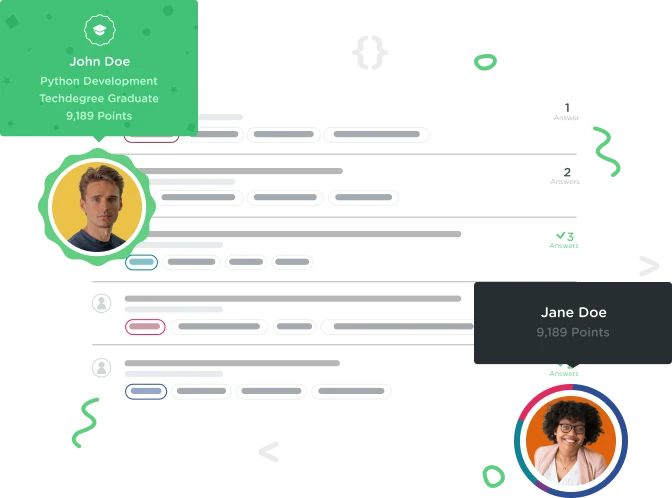
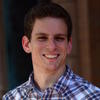
Jeff Lange
8,788 PointsCan someone help explain if/else to me?
For example, take this code:
var name = prompt("What is your name")
if (name) {
console.log("If block");
} else {
console.log("Else block");
}
The reason I'm confused is because it just stops at "if." If name. If name what? If name is Bob? If name is equal to 4? How does the console know whether to log "If block" or "Else block." Else what? I don't know how we defined "if" so how can we have an else?
Here's another one:
function doSomething (doit) {
var color = "blue",
number,
name = "Jim";
if(doit) {
color = "red";
number = 10;
console.log("Color in if(){}", color)
}
console.log("Color after if(){}", color)
}
"if(doit)". What does that even mean? It will run a console.log if doit does what?
4 Answers

sitkibagdat
27,311 PointsIf user doesn't enter a name, name variable will be undefined or empty string. These values are evaluate to false. So else block will run, otherwise if block will run.

sitkibagdat
27,311 Pointscode lines in if(true) always run, but code lines in if(name) run only if name is not false or undefined, zero, empty string, etc.
Here is a list of the values that are always evaluates to false:
- false
- 0 (zero)
- "" (empty string)
- null
- undefined
- NaN (a special Number value meaning Not-a-Number!)
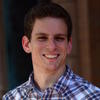
Jeff Lange
8,788 PointsSo another way of saying it would be "if name is true"? Can you write it in the code that way? i.e.
if (name = true)
And if (name)
is just shorthand?
What about in the second code box? In that one there is no alert for someone to put their name in or not (and thus cause a true or false value), so how does the computer determine whether "doit" is true or false?
And why is there no else statement? If there's no else statement does that just mean if the statement is false nothing happens? (i.e. there are no errors, just the code doesn't tell the computer to do anything)

mikescs
5,018 Points= is assignment operator for comparison you should use === or == (i am not going to describe it you can easily find it on the net). In this case you should use === but since if(name) will have the same effect it would be pointless to use ===.
The second box:
When you call the function. So when you call doSomething() without a parameter doIt then it will be false. Even if you call the function doSomething(false) that will have the same effect ..so the variables color,number,name will be (blue for color, undefined for number,jim for name).
If you call the function doSomething(1) (with any parameter basically) it gets set to true and if(doIt) evaluates as true hence the variables color,number get set.

sitkibagdat
27,311 PointsYes. A simple correction: it should be if (name == true) (double equal signs).
In the second example, if you pass a truthy value when calling doSomething() function, doit variable evaluates to true, otherwise false.
// If block will run
doSomething(true);
doSomething(5);
doSomething('any string');
// If block won't run
doSomething(false);
doSomething(0);
doSomething();
Else block is optional, so if you don't need you don't use it.
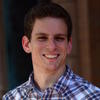
Jeff Lange
8,788 PointsOk, awesome, that makes sense.
You lost me with that codebox though. Where did that code come from? I'm assuming that's an illustration of what's going on behind the scenes? (i.e. if true it produces the given string in the console, whereas the empty parentheses in the "won't run" block represent the code not telling the computer to do anything). What are the 5 and the 0 for though?

sitkibagdat
27,311 PointsThese are possible examples(or techincally parameters, values, arguments, etc.) that you can use them with the doSomething() function that you wrote already. Please follow the lessons if you don't know anything about them, sorry.
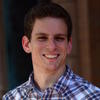
Jeff Lange
8,788 PointsOk gotcha, you were just using them as placeholders for any potential value. You could have easily used, 6, 110, x, etc. I thought maybe you were deriving them from somewhere.
Ok it makes sense now. Thank you for all your help! :)
And Mike S, thanks for your input as well, that was helpful. :)
Jeff Lange
8,788 PointsJeff Lange
8,788 PointsSo...it's saying if the original variable (var name) evaluates to true, it will run the if block. If it evaluates to false, it will run else?
Does it have to be entered as
if(name)
or could it be entered asif(true)
?