Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial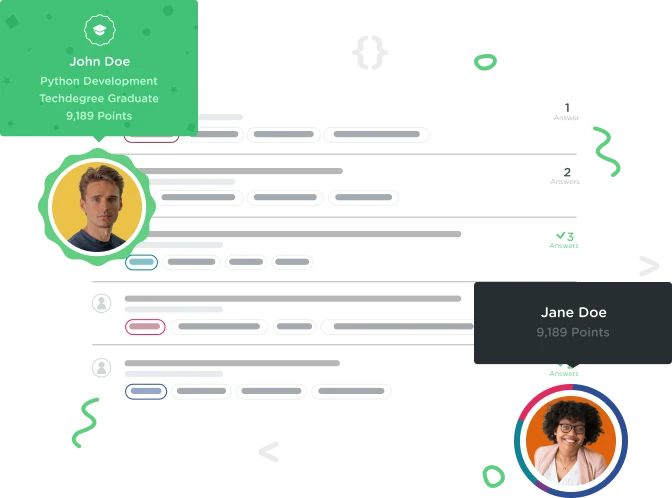

Adam Nwaozo
370 PointsCan someone help here I am getting an error message: Error:(48, 6) error: reached end of file while parsing
See my code:
public class FunFactsActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our view variables and assign views from the layout file
final TextView factlabel = (TextView) findViewById(R.id.textView3);
Button showFactButton = (Button) findViewById(R.id.button);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String[] facts = {
"Quote 1",
"Quote 2",
"Quote 3",
"Quote 4",
"Quote 5",
"Quote 6",
"Quote 7",
"Quote 8",
"Quote 9",
"Quote 10"};
//The button was clicked
String fact = "";
// Randomly Choose a fact
Random randomGenerator = new Random(); //Generates new random number
int randomNumber =randomGenerator.nextInt(facts.length);
fact = facts[randomNumber];
}
4 Answers
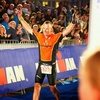
Steve Hunter
57,712 PointsYes, after that line, add this:
fact = facts[randomNumber]; // this is your code
} // make sure these next few lines look like this (comments unnecessary)
}; // closes the listener callback
} // closes onCreate()
The next line after that is:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
So, onCreateOptionsMenu
needs to be at the same level as the onCreate()
method your code is inside.
Make sure that all looks OK, then clear any errors at the end of the file due to there being too many closing braces.
Steve.
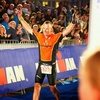
Steve Hunter
57,712 PointsThat's probably due to your brackets mismatched. You don't have the same number of opening brackets as you do closing ones. So, the compiler is expecting to find code, but reaches the end of the file instead.
Check your curly braces, that's probably where the problem lies.
Steve.

Adam Nwaozo
370 PointsWhere am I missing a curly brace?
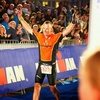
Steve Hunter
57,712 PointsIf you post the whole file, I can have a look.

Adam Nwaozo
370 Pointspublic class FunFactsActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our view variables and assign views from the layout file
final TextView factlabel = (TextView) findViewById(R.id.textView3);
Button showFactButton = (Button) findViewById(R.id.button);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String[] facts = {
"Quote 1",
"Quote 2",
"Quote 3",
"Quote 4",
"Quote 5",
"Quote 6",
"Quote 7",
"Quote 8",
"Quote 9",
"Quote 10"};
//The button was clicked
String fact = "";
// Randomly Choose a fact
Random randomGenerator = new Random(); //Generates new random number
int randomNumber = randomGenerator.nextInt(facts.length);
fact = facts[randomNumber];
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.fun_facts, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
{
return super.onOptionsItemSelected(item);
}
}
}
}
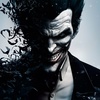
Alex Slye
4,427 PointsAndroid Studio is great at telling you where your curly braces match up. just click on one to see where its ending in your code. If this is your issue you should be able to find it quickly.
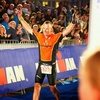
Steve Hunter
57,712 PointsYou're opening a brace on the OnClickListener
line, then another on the onClick()
line. That second one closes after the fact = facts[randomNumber];
line. Then you have a load of methods that should be in the same position within the class as onCreate()
.
I think if you add one curly brace after fact = facts[randomNumber];
, I think that gets everything on the right level.
Give that a go. And give me a shout if/when that fails. [EDIT: see additional answer below]
Steve.
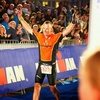
Steve Hunter
57,712 PointsHold on that - I think it needs an additional level as well as a semi-colon ... just checking.
Adam Nwaozo
370 PointsAdam Nwaozo
370 PointsI am not getting any errors in my code now but when I run it my arrays dont work or my button. :( see my code:
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYour code won't appear to do anything - you aren't setting anything on the screen to equal the fact you've generated.
Somewhere you need to have something like
factLabel.setText(fact);
Make sense?
Steve.