Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial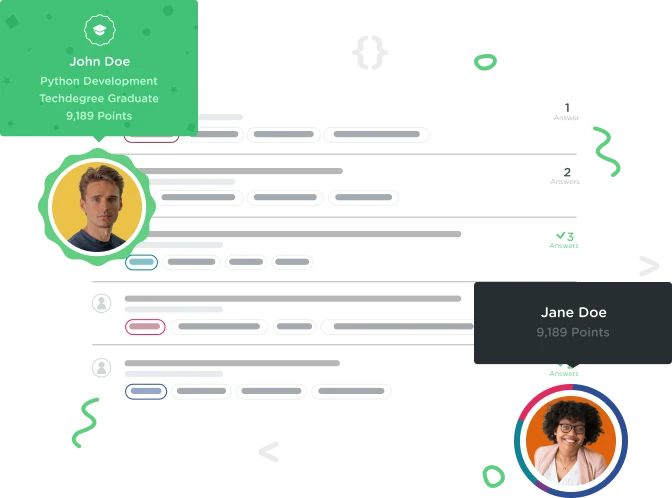
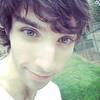
Alphonse Cuccurullo
2,513 PointsCan someone help me?
After your .sort! call, add an if statement. If rev is true, call reverse! on arr. Keep your numbers array and the puts statement so that you can see your work in action
def alphabetize(arr, rev=false)
arr.sort!
if rev == true
puts arr.reverse!
end
end
numbers = [1,4,5,6,7,8,55]
alphabetize(numbers)
puts numbers
Its telling me this " It looks like your method doesn't default to alphabetizing an array when it doesn't receive a second parameter."
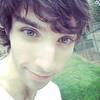
Alphonse Cuccurullo
2,513 PointsSo i did what you said and this popped up.
"It looks like your method doesn't default to alphabetizing an array when it doesn't receive a second parameter."

De Ming Liu
21,774 PointsLink to the problem you're having?

De Ming Liu
21,774 PointsOkay, so they actually want you to just "return" the answer in the function, not "puts" it. Use this:
def alphabetize(arr, rev=false)
arr.sort!
if (rev == true)
arr.reverse!
else
arr
end
end
numbers = [1, 2, 7, 3]
puts alphabetize(numbers)
Or this shorter version:
def alphabetize(arr, rev=false)
arr.sort!
if rev == true then arr.reverse! else arr end
end
numbers = ["c", "g", "h"]
puts alphabetize(numbers, true)
I did not include the "return" statement in the if/else statements because Ruby automatically returns the last statement, but you could add it if it helps you.
Cheers.
De Ming Liu
21,774 PointsDe Ming Liu
21,774 PointsYou didn't add the else after the if statement.
You want something like this, so it defaults to sorting the array if the 2nd parameter isn't entered (just like it explains):
Alternatively, he's a shorter way to do it: