Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial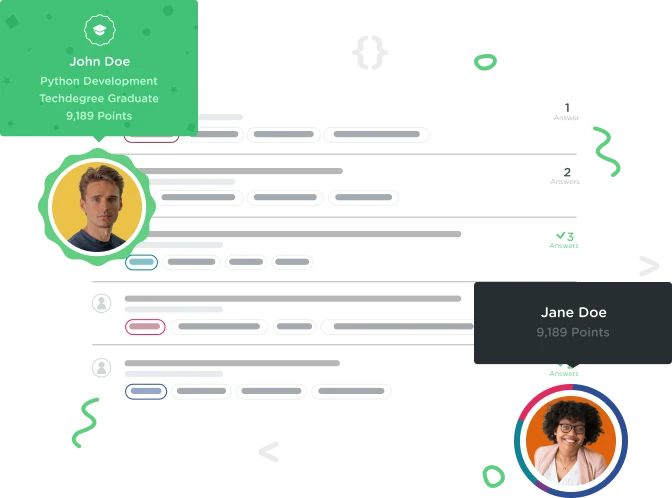

Ja'Nel Johnson
10,314 PointsCan someone help me make sense of Shared Preferences? I can't figure out the challenges.
Challenge 1: We'd like to persist the value of a CheckBox by using SharedPreferences. Start by declaring a constant for the file name of our preferences called PREFS_FILE. Then declare a constant for the key we'll require; call it KEY_CHECKBOX.
I went back to the video and followed along, but my answer is still wrong.
The error message keeps telling me to declare all constants.
Any suggestions?
import android.view.View;
import android.os.Bundle;
public class MainActivity extends Activity {
private static final STRING PREFS_FILE = "KEY_CHECKBOX";
public CheckBox mCheckBox;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mCheckBox = (CheckBox) findViewById(R.id.checkBox);
}
}
5 Answers
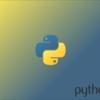
Kevin Faust
15,353 PointsHey Ja'Nel,
First thing I'd like to point out is that it should be "String" not "STRING"
Secondly, our PREFS_FILE constant should be equal to our packagename + .preferences. Remember that? It's just good naming convention.
Thirdly, we need a constant variable that holds the value of the state of our checbox. That is where you put KEY_CHECKBOX
In more visual terms look below:
private static final String PREFS_FILE = "com.packagename.preferences";
private static final String KEY_CHECKBOX = "KEY_CHECKBOX";
Hope that helped!
SharedPreferences was pretty tricky for me at first hehe
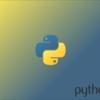
Kevin Faust
15,353 PointsHi Tina Bryan,
Context.MODE_PRIVATE has nothing to do with public/private variables. This is simply a paramater that the getSharedPreferences requires
From the android docs, "Use 0 or MODE_PRIVATE for the default operation, MODE_WORLD_READABLE and MODE_WORLD_WRITEABLE to control permissions."
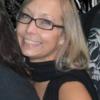
Tina Maddox
28,102 PointsThanks Kevin! Not sure why I didn't get this notice until now but thank you for the information!
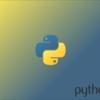
Kevin Faust
15,353 PointsHi Ja'Nel,
It should be
private SharedPreferences.Editor mEditor;
instead of just
private Editor mEditor;
Secondly your forgetting to put some things inside your getSharedPreferences("code should be in here")
mSharedPreferences = getSharedPreferences(PREFS_FILE, Context.MODE_PRIVATE);
Do you remember that? And then we initialize the mEditor variable by calling edit() on our mSharedPreferences
mEditor = mSharedPreferences.edit();
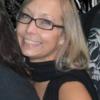
Tina Maddox
28,102 PointsHello Kevin (and Ja'Nel, whats up girl? :) ),
I am a little confused with the second line above:
mSharedPreferences = getSharedPreferences(PREFS_FILE, Context.MODE_PRIVATE);
When we declared it was 'public', so why is it 'private' here?
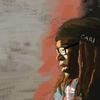
Carl Sergile
16,570 PointsHey having a hard time with this problem. On the second out of the four task. I've created the variables. I guess I just don't know where to plug it in. Here is my code:
import android.view.View;
import android.os.Bundle;
public class MainActivity extends Activity {
public CheckBox mCheckBox;
private static final String PREFS_FILE = "com.packagename.preferences";
private static final String KEY_CHECKBOX = "KEY_CHECKBOX";
private SharedPreferences mSharedPreferences;
private SharedPreferences.Editor mEditor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mCheckBox = (CheckBox) findViewById(R.id.checkBox);
mEditor = mSharedPreferences.edit();
mSharedPreferences = getSharedPreferences(PREFS_FILE, Context.MODE_PRIVATE);
}
}
UPDATED. Still showing a null error.
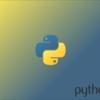
Kevin Faust
15,353 Pointsmy account is paused so i cant see what the question for part 2 is asking (if you could copy and paste it that would be helpful).
in terms of posting code here, just wrap your code in three black ticks with the language ur using. so for example:
```java
code```
btw you should do:
//swap order
mSharedPreferences = getSharedPreferences(PREFS_FILE, Context.MODE_PRIVATE);
mEditor = mSharedPreferences.edit();
because your calling edit() on mSharedPreferences when mSharedPreferences isnt initialized yet

Julie Carere
3,840 PointsOne thing I noticed was missing on the answers here that I found in the video, is to put private SharedPreferences mSharedPreferences;
So a complete correct solution is here:
import android.view.View;
import android.os.Bundle;
public class MainActivity extends Activity {
private SharedPreferences mSharedPreferences;
private SharedPreferences.Editor mEditor;
public CheckBox mCheckBox;
private static final String PREFS_FILE = "com.packagename.preferences";
private static final String KEY_CHECKBOX = "KEY_CHECKBOX";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mCheckBox = (CheckBox) findViewById(R.id.checkBox);
mSharedPreferences = getSharedPreferences(PREFS_FILE, Context.MODE_PRIVATE);
mEditor = mSharedPreferences.edit();
}
}
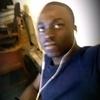
TERRENCE MAVHUNGA
6,053 Pointsthank you Kevin
Ja'Nel Johnson
10,314 PointsJa'Nel Johnson
10,314 PointsHey, Kevin!
Would you mind helping me with the next part? I'm following along but my answer isn't right.
Challenge 2: Now declare a SharedPreferences field named mSharedPreferences and an Editor field named mEditor. Then initialize both of these fields in the onCreate method.
Kevin Faust
15,353 PointsKevin Faust
15,353 Points//Edited code for markup