Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial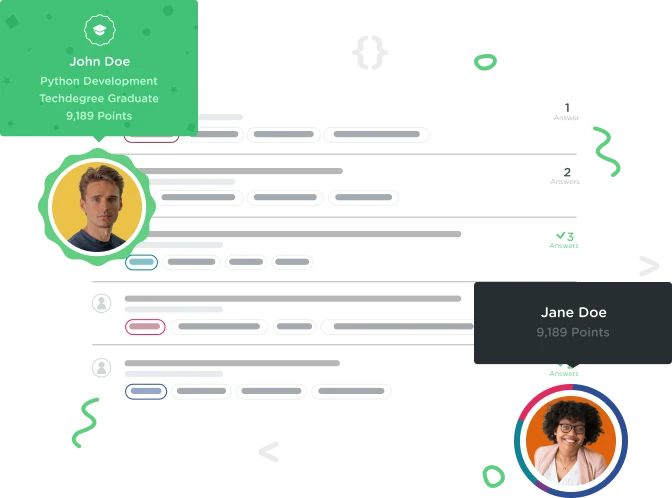
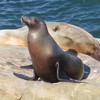
otarie
984 PointsCan someone help me solve this challenge? I think I'm having issues understanding the instructions!
The challenge is:
Add a score method to Game that takes a player argument that'll be either 1 or 2. Increase that player's value in self.current_score by 1. You'll need to adjust the index (i.e. player = 1 means self.current_score[0] needs to increase).
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self):
player = input("Enter a score of 1 or 2")
if player == 1 or player == 2:
self.current_score[0] += 1
return self.current_score
else:
return self.player
4 Answers

Michael Norman
Courses Plus Student 9,399 PointsSo, you need to create a method score that will be passed a player which will be a number 1 or 2. Based on which player is passesd in you should update that players score in the current_score list. Notice that
self.current_score = [0, 0]
So player 1 is index 0 and player 2 is index 1, a little confusing I know. There is no need to return an a value, you are just updating the current_score list in place. I have made some comments below to help guide you
class Game:
def __init__(self):
self.current_score = [0, 0]
# need to pass the player in. will be the integer 1 or 2
def score(self, player):
# no need to accept user input below. It wants the player passed into the method
#player = input("Enter a score of 1 or 2")
# if player 1 is passed in:
# update current_score for player 1. hint: what is the index of player 1 in current_score?
# if player 2 is passed in:
#update currrent_score for player 2
Hopefully this helps!

Emmet Lowry
10,196 PointsHi Michael i dont fully understand what you are saying would u mind breaking it down a bit more. Having trouble understanding what is being asked.

MUZ141140 Dennis Pomerai
10,215 Pointshie this is my answer, it passed
class Game: def init(self): self.current_score = [0, 0] def score(self, player): if player == 1: self.current_score[0] += 1 elif player == 2: self.current_score[1] += 1

Andrew Winkler
37,739 PointsNot that the rest of you are wrong, but there's a DRYer way ;)
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self, player):
self.current_score[player -1] += 1
To explain:
In this challenge you make a method inherits current_score via the self argument, but requires specificity regarding which player you are referring to via the player argument. Currently there are only 2 players: player 1 (self.current_score[0]) and player 2 (self.current_score[0]).
Your job is to increment the player's score at hand whose specificity is provided in the argument as either a 1 or a 2. Being that they aren't represented as such in the list/array you'll need to tack on a "-1" after referencing the player value. Then increment that players score by 1 ("+=1")