Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial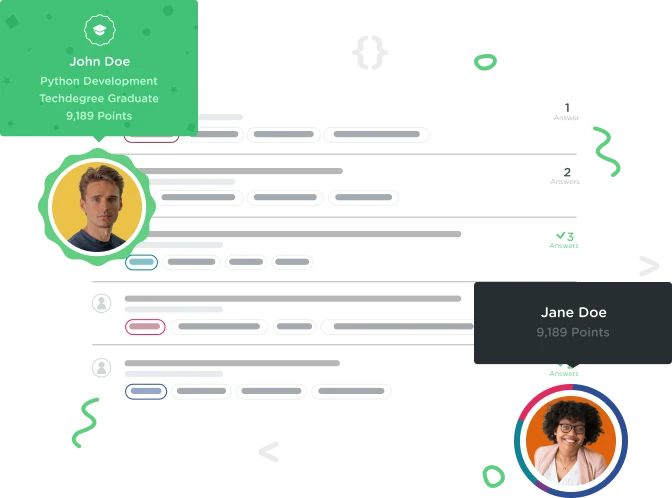

mohan Abdul
Courses Plus Student 1,453 PointsCan someone help me to rephrase the question? As i am not understanding what its asking.
"I'd like to be able to set the name attribute at the same
time that I create an instance. Can you add the code for doing that?
Remember, you'll need to override the init method."
What does it mean by ' i would like you to set the name attribute at the same time that i create an instance? what does it mean by overriding the __init__
method?
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
1 Answer
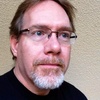
Chris Freeman
Treehouse Moderator 68,441 PointsYou are SO close. Set the attribute to the name
parameter:
def __init__ (self, name):
self.name = name
Post back if you need more help. Good luck!!!

mohan Abdul
Courses Plus Student 1,453 Pointsit says he would like to set the name attribute at the same time i create an instance. I thought that only instance is created when a class is used, so shouldn't it be '''self.name = Student()'''. thanks for your reply.
def __init__ (self, name):
self.name = name

mohan Abdul
Courses Plus Student 1,453 Pointscould you please try to rephrase the of what its asking? so i have a better understanding.
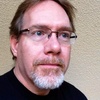
Chris Freeman
Treehouse Moderator 68,441 PointsThe better phrasing would be I would like you to set the name
attribute when the instance is initialized.
When an instance of the Student
class is created using Student()
, the __init__
method is run with self
pointing to the newly created instance. This makes the code reference self.some_attribute
apply to attributes on the new instance. The actual flow is:
- call
Student()
- this runs
Student.__new__
to create instance -
__new__
calls__init__
withself
pointing to new instance -
__init__
initializes the instance, creating attributes as desired, then exits, returningNone
. - execution returns to
__new__
method thatreturns
the initialized instance
Note __new__
is rarely discussed as it is a hidden part of the standard process. It later lessons you will see an example of the rare case where one might want to override the __new__
method. Spoiler: when inheriting from immutable classes like str
Post back if you need more help. Good luck!!!

mohan Abdul
Courses Plus Student 1,453 Pointswhat is __new__
? whats that referring? So what you are saying is that __init__
is run automatically, when Student() is called. thank you for you reply.

mohan Abdul
Courses Plus Student 1,453 Pointscan you clarify to me what is an instance, i always thought an instance is a result of something where a class is used.

mohan Abdul
Courses Plus Student 1,453 PointsChris Freeman, thanks for your reply in the latest thread, could you please try to answer the other questions in this thread. thanks for your support.
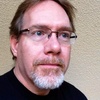
Chris Freeman
Treehouse Moderator 68,441 Pointswhat is __new__
? The method __new__
is automatically inherited from the class object
in every defined class.
So what you are saying is that __init__
is run automatically, when Student() is called. Exactly correct!!
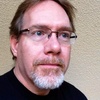
Chris Freeman
Treehouse Moderator 68,441 PointsAn class instance is the object created calling a class. That is, when parens () are used after a class name.
mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 Pointsthis is my blind guess.