Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial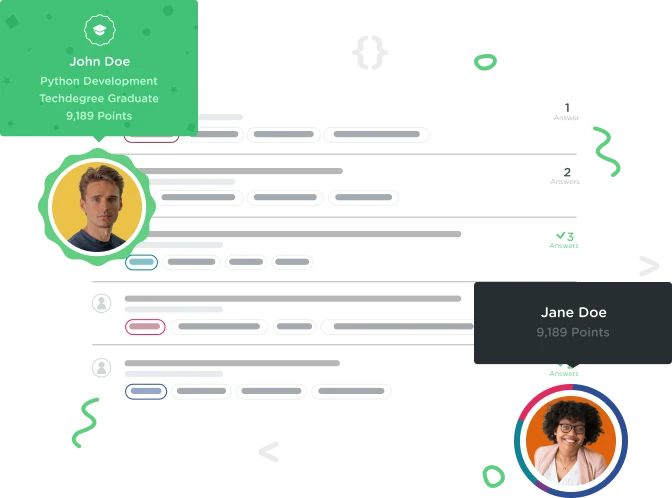

Tristan Gaebler
6,204 PointsCan someone help me understand the 'new' and 'this' keywords in java?
How do I use 'new' and 'this' properly? There purposes really confuse me. How are they used in terms of OOP?
2 Answers
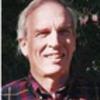
jcorum
71,830 PointsThe keyword new is used to create objects. When you write a class it either has a default constructor or one or more constructors you have written. When you create instances of the class (aka objects) you use those constructors. E.g.,
Student s1 = new Student("Tom", "Brown");
The first Student is the type, the s1 is the name you are giving the Student object that will be created, the = is the assignment operator, the new is the keyword that creates the object, the second Student is the constructor, and the ()s contain any parameters that need to be passed to the constructor to contract the object.
The keyword this means my:
public class Student {
String firstName;
String lastName;
public Student(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
//other methods
}
When values are passed as parameters to the constructor they are used to initialize the instance variables. this.firstName = firstName means assign the passed in value ("Tom") to my instance variable firstName.
Note that in most Treehouse videos the convention is to start instance variable names with m (for member variable). If you follow that convention, then your constructor for the Student class would look like this instead, and you wouldn't need to use this to disambiguate between the formal parameter and the instance (member) variable names.
public Student(String firstName, String lastName) {
mfirstName = firstName;
mlastName = lastName;
}
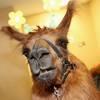
A X
12,842 PointsPerhaps a non-tech analogy would be making cookies. You could say by making say a batch of 24 cookies you're making 24 unique (new) instances of a cookie (in Java this would be new cookie, which means creating a cookie). Now let's say you want to eat one of the 24 cookies, how do you know which one to eat? You point to that one that has the most chocolate chips on it and say, "I'll eat this cookie!" (this cookie) However each new cookie has its own cool properties that you can assign...like one say, looks like a triangle. Each new instance can be referred to by the keyword this (which always refers to the object...in this case one of the cookies).
Also if baking doesn't suit you, you can think of puppies, kittens, or other baby animals. If you've ever been around a litter of baby animals you'll probably find yourself naming the infant animals based on their attributes. In say a litter of puppies, you might name one star from its Star shaped marking, Courage for her courageousness, or her brother Teddy for his soft fur. This is the same concept. New puppy instance, and this.puppy = 'Teddy', this.puppy = 'Courage', this.puppy='Star';